In the past, we have used cookies to save data generated by user, on the client side. But there have been multiple issues with cookies, mainly due to its insecure nature. Because of these issues, users browse the web by disabling cookies or deleting them from time to time.
In HTML5, a new standard web storage was created called as LocalStorage and sessionStorage. Typically these are really useful to save data in cases where:
- Browser Crashes accidently.
- User closes browser accidently
- Internet connection lost.
LocalStorage, object provides the following methods:
- setItem: It takes two parameters, the first is the label for the data using which it will be identified in the LocalStorage, this is also called as key. The second parameter is data itself. This data must be a string. So if JavaScript object is to be stored in the LocalStorage, then it must be converted into JSON format. Using this method the data will be locally stored with the user.
- getItem: This is a natural opposite to setItem, because if the data is stored locally, it will be retrieved back. This method accepts the key of the data stored into the LocalStorage.
- removeItem: Once the data is retrieved and its no more required, then it must be removed. This method accepts the key of the data and removes the data from the LocalStorage.
- clear: This clears the LocalStorage.
Step 1: Open Visual Studio 2012 or any Web Editor of your choice and create a new Blank HTML page. In this project add a new HTML page, name it as ‘H5_LocalStorage.html’. Add a new folder in the project, name it as ‘MyScripts’.
Step 2: Design the html page with following Markup:
<table style="width:100%;" id="tbl">
<tr>
<td>Person Id:</td>
<td>
<input id="txtpid" type="text" class="c1"/></td>
</tr>
<tr>
<td>Person Name:</td>
<td>
<input id="txtpname" type="text" class="c1" /></td>
</tr>
<tr>
<td>Address:</td>
<td>
<textarea id="txtpaddr" class="c1"></textarea></td>
</tr>
<tr>
<td>City:</td>
<td>
<input id="txtpcity" type="text" class="c1"/></td>
</tr>
<tr>
<td>Email:</td>
<td>
<input id="txtpemil" type="email" class="c1" /></td>
</tr>
<tr>
<td>Age:</td>
<td>
<input id="txtpage" type="text" class="c1"/></td>
</tr>
<tr>
<td>Occupation:</td>
<td>
<input id="txtpoccup" type="text" class="c1"/></td>
</tr>
<tr>
<td>Mobile No:</td>
<td>
<input id="txtpmobile" type="tel" class="c1"/></td>
</tr>
<tr>
<td>Gender:</td>
<td>
<select id="lstpgender" class="c1">
<option>Male</option>
<option>Female</option>
</select>
</td>
</tr>
<tr>
<td> </td>
<td> </td>
</tr>
<tr>
<td><input id="btnsave" type="button" value="Save" /></td>
<td><input id="btnclear" type="button" value="Clear" /></td>
<td><input id="btnclearstorage" type="button" value="Clear Storage" /></td>
</tr>
</table>
<div id="dvcontainer">
</div>
In the above markup, the <div> with id as ‘dvcontainer’ will be used to generate a Table dynamically to display data from the LocalStorage.
Step 3: In the MyScripts folder add a new JavaScript file and name it as ‘LocalStorageLogic.js’. In the file we will add logic for storing data in the LocalStorage. The code in the file is as below:
(function () {
//The Person Object used to store data in the LocalStorage
var Person = {
Id: 0,
Name: "",
Address: "",
City:"",
Email: "",
Age:0,
Occupation: "",
MobileNo: "",
Gender:""
};
//JavaScript object containing methods for LocalStorage management
var applogic = {
//Clear All Entries, by reading all elements having class as c1
clearuielements: function () {
var inputs = document.getElementsByClassName("c1");
for (i = 0; i < inputs.length; i++) {
inputs[i].value = "";
}
},
//Save Entry in the Localstorage by eading values entered in the
//UI
saveitem: function () {
var lscount = localStorage.length; //Get the Length of the LocalStorage
//Read all elements on UI using class name
var inputs = document.getElementsByClassName("c1");
Person.Id = inputs[0].value;
Person.Name = inputs[1].value;
Person.Address = inputs[2].value;
Person.City = inputs[3].value;
Person.Email = inputs[4].value;
Person.Age = inputs[5].value;
Person.Occupation = inputs[6].value;
Person.MobileNo = inputs[7].value;
Person.Gender = inputs[8].value;
//Convert the object into JSON ans store it in LocalStorage
localStorage.setItem("Person_" + lscount, JSON.stringify(Person));
//Reload the Page
location.reload();
},
//Method to Read Data from the local Storage
loaddata: function () {
var datacount = localStorage.length;
if (datacount > 0)
{
var render = "<table border='1'>";
render += "<tr><th>Id</th><th>Name</th><th>Address</th><th>City</th>” + “<th>Email</th><th>Age</th><th>Occupation</th><th>MobileNo</th>” +<th>Gender</th></tr>";
for (i = 0; i < datacount; i++) {
var key = localStorage.key(i); //Get the Key
var person = localStorage.getItem(key); //Get Data from Key
var data = JSON.parse(person); //Parse the Data back into the object
render += "<tr><td>" + data.Id + "</td><td>" + data.Name + " </td>";
render += "<td>" + data.Address + "</td>";
render += "<td>" + data.City + "</td>";
render += "<td>" + data.Email + "</td>";
render += "<td>" + data.Age + "</td>";
render += "<td>" + data.Occupation + "</td>";
render += "<td>" + data.MobileNo + "</td>";
render += "<td>" + data.Gender + "</td></tr>";
}
render+="</table>";
dvcontainer.innerHTML = render;
}
},
//Method to Clear Storage
clearstorage: function () {
var storagecount = localStorage.length; //Get the Storage Count
if (storagecount > 0)
{
for (i = 0; i < storagecount; i++) {
localStorage.clear();
}
}
window.location.reload();
}
};
//Save object into the localstorage
var btnsave = document.getElementById('btnsave');
btnsave.addEventListener('click', applogic.saveitem, false);
//Clear all UI Elements
var btnclear = document.getElementById('btnclear');
btnclear.addEventListener('click', applogic.clearuielements, false);
//Clear LocalStorage
var btnclearstorage = document.getElementById('btnclearstorage');
btnclearstorage.addEventListener('click', applogic.clearstorage, false);
//On Load of window load data from local storage
window.onload = function () {
applogic.loaddata();
};
})();
The above code has the following specifications:
- The ‘Person’ object which defines properties for containing Person information.
- The ‘applogic’ object contains the following methods:
saveitem:
- This method first reads all input elements from the html page using the class name.
- Then pass that data entered into these UI elements into the Person object.
- The Person object is then converted into the JSON string and stored into LocalStorage using setItem method.
- The page is then reloaded. This is because data stored must be displayed on the UI.
- This method is used to read data from the LocalStorage in to the Table.
- This method first reads the length of the data stored into the LocalStorage.
- If this is greater than zero, then each entry from the LocalStorage is read using a Key.
- The data is then converted back to JavaScript object using JSON.parse() method.
- Finally each property from the JavaScript object is displayed by generating HTML table dynamically.
Finally in the code we subscribe to the click event of the buttons using addEventListener method and pass methods from the above applogic object to it. The window.onload event is used to load data from the LocalStorage.
Run the application in the Chrome browser or any browser that supports the option of viewing
browser resources. Here are some screen-shots UI when the page is loaded for the first time:
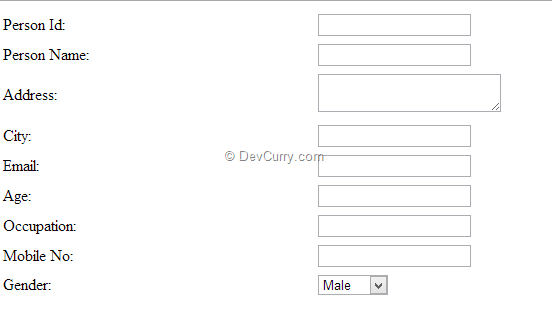
Add data in each TextBox and click on ‘Save’ button. Similarly enter 2-3 records:
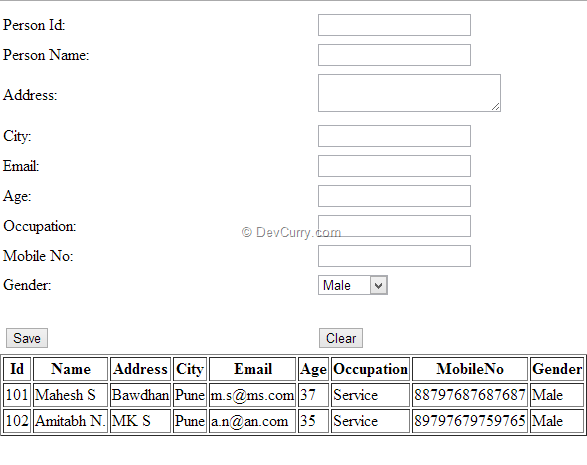
You can find the data as below using F12 on Chrome
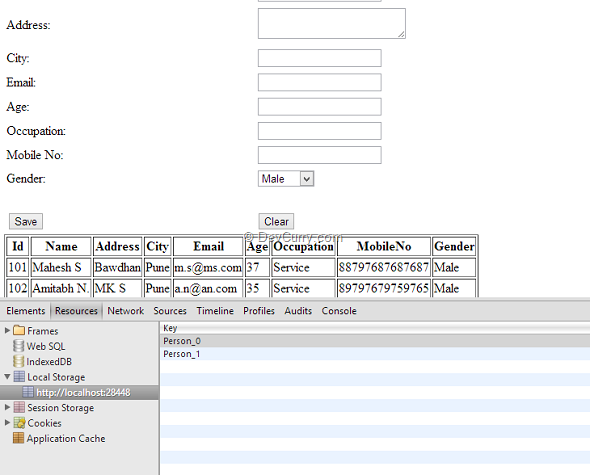
The data can be cleared by clicking on the ‘Clear Storage’ button.
Conclusion: The Web Storage provided with HTML5 helps to manage data in a web page in an organized manner in a real world Line-of-Business (LOB) application.
Tweet
13 comments:
Hi,
I am using your step for local storage.Nothing happened when running html page on chrome.
Can you tell me in brief what is the actual issue.
in your html file add this line:
script src="MyScripts/LocalStorageLogic.js"
Change the folder file name to fit your naming conventions.
Great Job!
I am working on a proposal and the RFP needs are to have a web application with offline capabilities. The Offline capabilities are required for an extensive data entry process where there are about 5 Chapters, tons of Grids and hundreds of Questions. This data entry process needs to be implemented through a web interface ensuring that the users from the countries who have unreliable internet connectivity can work offline for most part of the data entry work (may be for days and weeks) and once they are ready with the data entered, then the application should be able to sync it back to the main database....
Do you think HTML5 is mature enough to handle such complex and high volume offline data??
thanks and regards
Sumeet
Hi, I have run your code on browser. but when i click save there is nothing display out. So how can I know whether my data is save or not save?
I had the same problem.
There is missing code to tell the html file about the .js file.
Just before the div id="dvcontainer" line in the H5_LocalStorage.html file enter this line:
script src="MyScripts\LocalStorageLogic.js" /script
add the Greater than and Less than characters.
That made mine work.
John Wilson
I also get extraneous characters above the table where the entries are displayed.
They are: "+""+
I am not sure where they come from.
The extraneous characters are on line 50 of LocalStorageLogic.js between the tags.
after adding Just before the div id="dvcontainer" line in the H5_LocalStorage.htm
Error is "Object doesn't support this property or method" line 87 char 1,can you please resolve it
hi how to delete particular item from local storage?
I have used your code. Using loaddata() function m displaying item along with button. But Not getting how to delete particular item. Please help me out..
0x800a01b6 - JavaScript runtime error: Object doesn't support property or method 'addEventListener'
How can I do now?
hey i am using webstorm and i run this program and it doesnt do anything can anyone help me out.
Post a Comment