Backbone Basics
Backbone.js is a lightweight library for structuring client side code. This easily helps to decouple concerns in the application. Typically, this library is used to build SPA’s. The advantage of using backbone is that it provides lightweight data structuring mechanism using model and collection and helps to use these in generated Views. This is very important for designing dynamic applications using JavaScript. You can get detailed information from http://backbonejs.org/.There are some important features provided by Backbone as discussed below:
Model:
These are used to contain data for the application and logic around this data. This is defined by extending the Backbone.Model as below:
var Product=Backbone.Model.extend({
//your properties for Model goes here
});
Model is the major important declaration for the Data-Driven Web applications. In real world application development, this comes from external Web/WCF services or WEB API. The data store is in the form of JSON.
Views:
This contains the logic behind presenting the Model to the user. The data is presented using JavaScript Templating e.g. jQuery tmpl (outdated), Underscore.js, Moustache.js etc. The View can be created as below:
var productView = Backbone.View.extend({});
The important part of the view is the render method which is actually responsible for presenting the Model data to user using the Templates.
One important property of the view is el. This is the reference to the DOM element and is used to compose the element’s content and render them into DOM at once. This helps for fast rendering.
Collection:
These are the model sets. These are defined as below:
var productCollection = Backbone.Collection.extend({
model: <name of the model>
});
Routers:
These provide a way to user to connect to the URL generally using hash (#) fragment.
e.g.
http://myserver.com/#info
It is defined as below:
var MyRouter = Backbone.Router.extend({
routes:{
“info” : “ProductInfo”
},
ProductInfo :function(){
}
});
In the following demo we will implement a simple implementation of Model, Collection and View.
Step 1: Although you can use any Web Editor of your choice, we will use Visual Studio which also contains a free express edition. Open Visual Studio 2012/2013 and create a new Web Application. In this application using NuGet package, add jQuery and Backbone scripts.
Step2: In the project, add a new JavaScript file, name it as Models.js and in this file refer the scripts as below:
/// <reference path="../Scripts/backbone.min.js" />
/// <reference path="../Scripts/underscore.min.js" />
backbone.js library has a dependency on underscore.js, so when you add backbone in the current project using NuGet package, Underscore.js will gets added automatically.
Now define the Model as below:
var Employee = Backbone.Model.extend({
defaults: {
EmpNo: 0,
EmpName: "",
DeptName: "",
Designation:"",
Salary:0.0
}
});
The model has Employee properties with default values set for it. Define collection as below:
//Declaring Employee Collection
var EmployeesCollection = Backbone.Collection.extend({
model: Employee
});
Define an instance of the Collection as below and add a Model in it:
//The Collection Instance
var Employees = new EmployeesCollection();
Employees.add(new Employee({
'EmpNo': 101, 'EmpName': "Mahesh",
'DeptName': "D1", 'Designation': "TL", 'Salary': 23400
}));
Employees.add(new Employee({
'EmpNo': 102, 'EmpName': "Sachin",
'DeptName': "D1", 'Designation': "SM", 'Salary': 32400
}));
Employees.add(new Employee({
'EmpNo': 103, 'EmpName': "Amitabh",
'DeptName': "D2", 'Designation': "PD", 'Salary': 33400
}));
Employees.add(new Employee({
'EmpNo': 104, 'EmpName': "Amit",
'DeptName': "D2", 'Designation': "SM", 'Salary': 43400
}));
Employees.add(new Employee({
'EmpNo': 105, 'EmpName': "Rupesh",
'DeptName': "D3", 'Designation': "DP", 'Salary': 23400
}));
Employees.add(new Employee({
'EmpNo': 106, 'EmpName': "Bhalchandra",
'DeptName': "D4", 'Designation': "SE", 'Salary': 43400
}));
Task 3: In this project add a new HTML page, name it as HT_Collection_Rendering_View.html. In this add the script references as below:
<script src="Scripts/jquery-2.0.3.min.js"></script>
<script src="Scripts/underscore.min.js"></script>
<script src="Scripts/backbone.min.js"></script>
<script src="Backcone_Custom_Scripts/Models.js"></script>
Step 4: In the <body> tag, add the following script for the View.
<script type="text/javascript">
//Define View
var EmpView = Backbone.View.extend({
//The HTML Element
el: 'body',
//Render View
render: function () {
var viewHtml = '<table border="1">';
viewHtml += "<tr><td>EmpNo</td><td>EmpName</td><td>DeptName</td><td>Designation</td> <td>Salary</td></tr>";
//Iterate through the collection
_.each(this.collection.models, function (m, i, l) {
var empRecHtml = '<tr><td>' + m.get('EmpNo') + '</td><td>' + m.get('EmpName') + '</td><td>' + m.get('DeptName') + '</td><td>' + m.get('Designation') + '</td><td>' + m.get('Salary') + '</td></tr>';
viewHtml += empRecHtml;
});
viewHtml +='</table>'
//Set the View
$(this.el).html(viewHtml);
}
});
//Thew View Instance and Passing Collection to it
var eView = new EmpView({
collection: Employees
});
//Render the View
eView.render();
</script>
The above script defines View object, the element el here defines the ‘body’ means the DOM will be inserted in <body> tag. The render method defines html table. In this html table values from the collection are shown by iterating through the collection using _.each(); this function is present in underscore.js framework. The get() method is used to retrieve values for the model property. Finally view object is created with the name eView and the Employees collection is passed to it and then the render() method is called.
Step 5: Now view the page in browser and data from the collection will be presented as below:
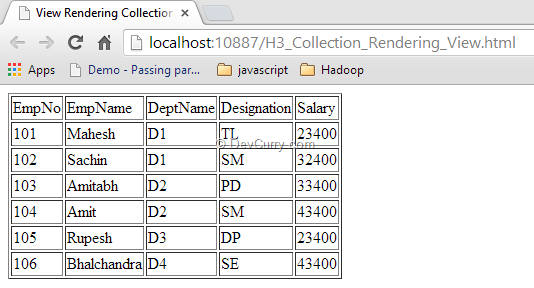
Conclusion: Backbone framework provides an easy mechanism to define Models, Collection, Views separately so that decoupled client-side code for web applications can be easily implemented.
Tweet
2 comments:
good one
Post a Comment