For this article, the Sql Server Database server is used with ‘Company’ as database and ‘EmployeeInfo’ as the table. The script is as below:
CREATE TABLE [dbo].[EmployeeInfo](
[EmpNo] [int] IDENTITY(1,1) NOT NULL,
[EmpName] [varchar](50) NOT NULL,
[Salary] [decimal](18, 0) NOT NULL,
[DeptName] [varchar](50) NOT NULL,
[Designation] [varchar](50) NOT NULL,
CONSTRAINT [PK_EmployeeInfo] PRIMARY KEY CLUSTERED
(
[EmpNo] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF,
IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON)
ON [PRIMARY]
) ON [PRIMARY]
GO
Step 1: Open VS2012 and create a MVC application. Name it as ‘MVC_jQuery_AutoComplete’. In this project, add the model for the above table using Ado.NET EF. After completing the wizard for ADO.NET, the entity will be displayed as below:
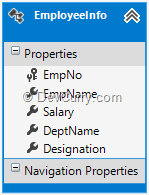
Step 2: Build the Project. Now to add a WEB API Controller, right- click on the controller folder and add the new WEB API controller based upon the ADO.NET EF. This will generate Read/Write API Actions as below:
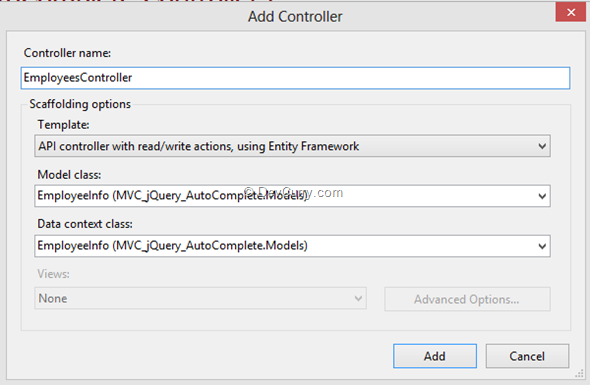
This step will add a new WEB API class with Read and Write methods. These methods perform communication using JSON.
Step 3: In the project, add a new empty EmployeeInfoController. This controller will only have the Index method. We are doing this so that we can add a new Empty Index View.
Step 4: Add a new Index Empty view in the project by right clicking on the Index method of the EmployeeInfoController class. In this View, add the HTML control as below:
<span>Enter The Employee Name Here:</span>
<input type="text" id="txtempname" />
Step 5: In the Scripts folder add below script files, these you can get from www.jquery.com and www.jqueryui.com.
- jquery-1.8.2.js
- jquery-ui-1.9.0.js
- jquery-ui-1.9.0.min.js
- jquery-ui-1.9.0.custom.css
<link href="~/css/jquery-ui-1.9.0.custom.css" rel="stylesheet" />
<script src="~/Scripts/jquery-1.8.2.js"></script>
<script src="~/Scripts/jquery-ui-1.9.0.min.js"></script>
Step 7: Write the below style in the Index.cshtml, this defines the appearance for the Auto-Complete:
<style>
.ui-autocomplete {
max-height: 100px;
overflow-y: auto;
}
.ui-autocomplete {
height: 100px;
}
</style>
Step 8: Add the following script shown here. This makes an AJAX call to the WEB API (your port may be different), the JSON data is received and then it is converted into an Array. This array is then passed to the source parameter of the autocomplete method defined for the textbox.
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
type: 'GET',
url: "http://localhost:49601/api/Employees",
datatype: "application/json; charset=utf-8",
success: function (data) {
var Names = [];
Names = getDataInArray(data);
$("#txtempname").autocomplete({
source: Names,
});
}
});
//Method to Convert JSON Response into an Array
function getDataInArray(array) {
var names = [];
$.each(array, function (i, d) {
names.push(d.EmpName);
});
return names;
}
});
</script>
Step 9: Run the application and navigate to the EmployeeInfo/Index, you will see the textbox. Add some text into it. If it matches with the data returned from the WEB API call, it will be displayed in the AutoComplete area as below:
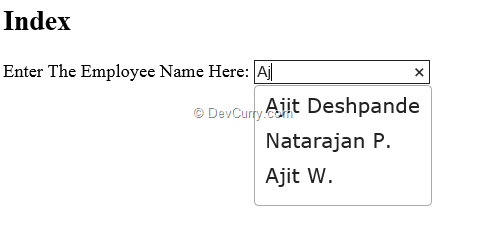
Conclusion
Ideally we should restrict the number of rows returned to Top 10 or 20 results for performance considerations. It’s pretty easy to use the jQuery UI plugin and add AutoComplete behavior to a text box. Overall jQuery UI is a powerful tool kit to have in one’s web development portfolio.
Download the entire source code
Tweet
4 comments:
you explain so nicely :) Great!
Well done, thanks.
Thanks for this. I've found many others but your explanation got me working!
thanks a lot...it works for me,thank YOU
Post a Comment