WPF XAML has huge capabilities for effective UX development. The following code shows how to display multi-column data values for ComboBox in WPF
C#
using System.Windows;
using System.Collections.ObjectModel;
namespace WPF_MultiColumnCombobox
{
/// <summary>
/// Interaction logic for Window1.xaml
/// </summary>
public partial class Window1 : Window
{
ObservableCollection<Empl> _emp = new ObservableCollection<Empl>();
public Window1()
{
InitializeComponent();
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
_emp.Add(new Empl() {EmpNo = 101, EmpName = "Mahesh", DeptName = "IT" });
_emp.Add(new Empl() {EmpNo = 102, EmpName = "Ajay", DeptName = "IT" });
_emp.Add(new Empl() {EmpNo = 103, EmpName = "Rahul", DeptName = "IT" });
_emp.Add(new Empl() {EmpNo = 104, EmpName = "Pradnya", DeptName = "HR" });
_emp.Add(new Empl() {EmpNo = 105,EmpName = "Abhijeet",DeptName = "ITSF" });
_emp.Add(new Empl() {EmpNo = 106, EmpName = "Keshav", DeptName = "MKTG" });
_emp.Add(new Empl() {EmpNo = 107,EmpName = "Prasanna",DeptName = "MKTG" });
_emp.Add(new Empl() {EmpNo = 108, EmpName = "Shailesh", DeptName = "BH" });
_emp.Add(new Empl() {EmpNo = 109,EmpName = "Sidhhart",DeptName = "ITSF" });
_emp.Add(new Empl() {EmpNo = 1010, EmpName = "Kaipl", DeptName = "AI" });
this.DataContext = _emp;
}
}
public class Empl
{
public int EmpNo { get; set; }
public string EmpName { get; set; }
public string DeptName { get; set; }
}
}
XAML Code
<Window x:Class="WPF_MultiColumnCombobox.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Window1" Height="300" Width="560" Loaded="Window_Loaded" >
<Window.Resources>
<Style x:Key="txtStyle" TargetType="{x:Type TextBlock}">
<Setter Property="TextAlignment" Value="Center"></Setter>
</Style>
<DataTemplate x:Key="EmpKey">
<Grid Height="50" Width="160" ShowGridLines="True">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="40"></ColumnDefinition>
<ColumnDefinition Width="60"></ColumnDefinition>
<ColumnDefinition Width="50"></ColumnDefinition>
</Grid.ColumnDefinitions>
<TextBlock Grid.Column="0" Text="{Binding EmpNo}"
Style="{StaticResource txtStyle}"></TextBlock>
<TextBlock Grid.Column="1" Text="{Binding EmpName}"
Style="{StaticResource txtStyle}"></TextBlock>
<TextBlock Grid.Column="2" Text="{Binding DeptName}"
Style="{StaticResource txtStyle}"></TextBlock>
</Grid>
</DataTemplate>
<Style TargetType="{x:Type ComboBoxItem}">
<Style.Triggers>
<DataTrigger Binding="{Binding Path=DeptName}" Value="IT">
<Setter Property="Background" Value="White"></Setter>
</DataTrigger>
<DataTrigger Binding="{Binding Path=DeptName}" Value="HR">
<Setter Property="Background" Value="Blue"></Setter>
</DataTrigger>
<DataTrigger Binding="{Binding Path=DeptName}" Value="ITSF">
<Setter Property="Background" Value="Gray"></Setter>
</DataTrigger>
<DataTrigger Binding="{Binding Path=DeptName}" Value="BH">
<Setter Property="Background" Value="DarkGray"></Setter>
</DataTrigger>
<DataTrigger Binding="{Binding Path=DeptName}" Value="AI">
<Setter Property="Background" Value="Blue"></Setter>
</DataTrigger>
<DataTrigger Binding="{Binding Path=DeptName}" Value="MKTG">
<Setter Property="Background" Value="DarkBlue"></Setter>
</DataTrigger>
</Style.Triggers>
</Style>
</Window.Resources>
<Grid>
<ComboBox Height="38" Margin="0,27,0,0"
Name="comboBox1"
VerticalAlignment="Top" ItemsSource="{Binding}"
ItemTemplate="{StaticResource EmpKey}"
IsSynchronizedWithCurrentItem="True"
HorizontalAlignment="Left" Width="200">
</ComboBox>
</Grid>
</Window>
The above .Xaml defines DataTemplate which represents the way data will be displayed. We also have the data styles defined based upon the values that will be displayed in the ComboBox. For eg: When the value of the DeptName is “HR”, the color of the row is Blue and so on.
Here’s the output:
Clicking on the ComboBox, you get an output similar to the following:
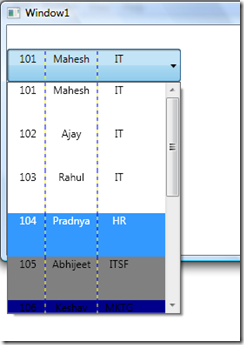