While using Silverlight for developing Line-of-Business applications, the DataForm control is recommended for performing DML operations. This control provides data pagination, insert, and update and delete operations on the records. This control provides ItemsSource property which accepts collection type as an input e.g. ObservableCollection. But while performing Insert and Update operations, it is important to perform Data Validations. In Silverlight, we have been provided with the IDataErrorInfo and INotifyDataErrorInfo interfaces for Data Validations. At the same time, we have been provided with DataAnnotation features using which properties in the source class, can be validated. In the code below, I have demonstrated the use of data annotation for data validations.
Step 1: Open VS2010 and create a new Silverlight application, name it as ‘SL4_DataForn_DataAnnotation’.

Step 3: Write the following class with Data Annotation attributes:
public class EmployeeInfo {
private int _EmpNo;
[Display(Name = "EmpNo", Description = "Enter Employee No.")]
[Required(ErrorMessage = "Field Cannot be Left Blank")]
public int EmpNo
{
get { return _EmpNo; }
set
{
_EmpNo = value;
}
}
private string _EmpName;
[Display(Name = "EmpName", Description = "Enter Employee Name")]
[Required(ErrorMessage = "Field Cannot be Left Blank")]
public string EmpName
{
get { return _EmpName; }
set
{
_EmpName = value;
}
}
private string _DeptName;
[Display(Name = "DeptNo", Description = "Enter Department No.")]
[Required(ErrorMessage = "Field Cannot be Left Blank")]
public string DeptName
{
get { return _DeptName; }
set
{
_DeptName = value;
}
}
private int _Salary;
[Display(Name = "Salary", Description = "Enter Salary.")]
[Required(ErrorMessage = "Field Cannot be Left Blank")]
public int Salary
{
get { return _Salary; }
set
{
_Salary = value;
}
}
private string _Email;
[Display(Name = "Email", Description = "Enter Email.")]
[Required(ErrorMessage = "Field Cannot be Left Blank")]
[RegularExpression(@"\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*",
ErrorMessage = "Invalid E-Mail ID")]
public string Email
{
get { return _Email; }
set
{
_Email = value;
}
}
private int _Age;
[Display(Name = "Age", Description = "Enter Age.")]
[Required(ErrorMessage = "Field Cannot be Left Blank")]
[Range(23, 65, ErrorMessage = "Not a Valid Range")]
public int Age
{
get { return _Age; }
set
{
_Age = value;
}
}
}
public class EmployeesInfoCollection : ObservableCollection<EmployeeInfo>
{
public EmployeesInfoCollection()
{
Add(new EmployeeInfo() { EmpNo = 101, EmpName = "Mahesh Sabnis", DeptName = "CTD", Salary = 56000, Email = "mahesh.sabnis@s***h.com", Age = 33 });
Add(new EmployeeInfo() { EmpNo = 102, EmpName = "Ravi Tambade", DeptName = "CTD", Salary = 90000, Email = "ravi.tambade@s***h.com", Age = 35 });
Add(new EmployeeInfo() { EmpNo = 103, EmpName = "Vikram Pendse", DeptName = "SCS", Salary = 45000, Email = "vikram.pendse@s***h.com", Age = 28 });
Add(new EmployeeInfo() { EmpNo = 104, EmpName = "Natarajan Padallalu", DeptName = "CTD", Salary = 34000, Email = "saket,karnik@s***h.com", Age = 30 });
Add(new EmployeeInfo() { EmpNo = 105, EmpName = "Kiran Patange", DeptName = "CTD", Salary = 20000, Email = "manish.sharma@s***h.com", Age = 27 });
Add(new EmployeeInfo() { EmpNo = 106, EmpName = "Sachin Nimbalkar", DeptName = "CTD", Salary = 34000, Email = "varun.lokhande@s***h.com", Age = 27 });
Add(new EmployeeInfo() { EmpNo = 107, EmpName = "Vishal Dulare", DeptName = "CTD", Salary = 39000, Email = "abhijeet.gole@s***h.com", Age = 29 });
Add(new EmployeeInfo() { EmpNo = 108, EmpName = "Amitabh Ninawe", DeptName = "CTD", Salary = 20000, Email = "sachin.shukre@s***h.com", Age = 32 });
Add(new EmployeeInfo() { EmpNo = 109, EmpName = "Sachin Shukra", DeptName = "CTD", Salary = 34000, Email = "jyoti.ghadge@s***h.com", Age = 30 });
Add(new EmployeeInfo() { EmpNo = 110, EmpName = "Aniket Kirkole", DeptName = "SYS", Salary = 90000, Email = "aniket.kirkole@s***h.com", Age = 37 });
}
}
The EmployeeInfo class contains properties with the data validations for every property. Classes used for validation attributes are as below:
- Required: Specifies the value is must.
- RegularExpression: Specifies the regular expression using which the input data can be verified.
- Range: Specifies the minimum to maximum range for the data to be entered.
Step 4: Open MainPage.xaml and add the DataForm control as shown below:
Note: Drag-Drop it on the designer because this is not available by default in the toolbox. The xaml code for configuring the DataForm is as below:
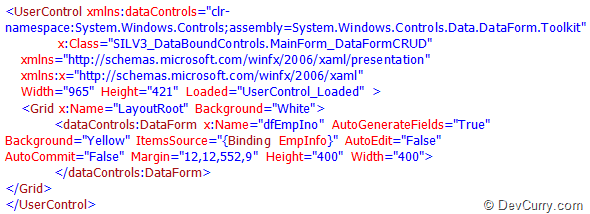
Note that the DataForm control sets the following properties:
- AutoEdit: When set to False, provides the ‘Edit’ button on the DataFrom Pager control.
- AutoCommit: When set to false, the button ‘Ok’ is generated to save changes made to the collection using DataFrom field.
Step 5: In the MainPage.xaml.cs write the following code:
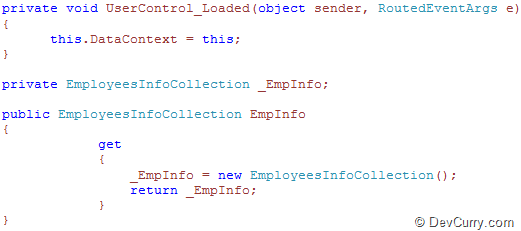
The above code defines a public property ‘EmployeeInfoCollection’ which is used to bind with the DataForm control on UI.
Step 6: Run the application, the result will be as below:
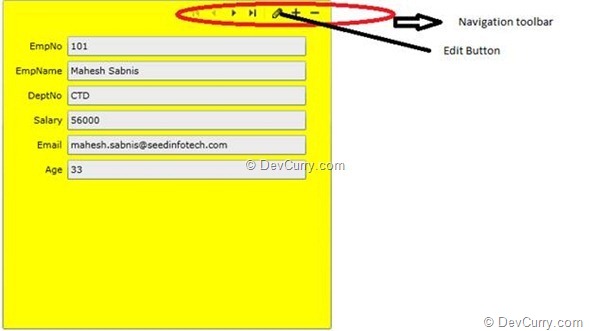
Click on the Edit Button and the record will be enabled. Change the Age and click on the ‘OK’ button that gets generated. Make sure that the Age you enter is more than 100 for the validation to trigger. The result will be as below:
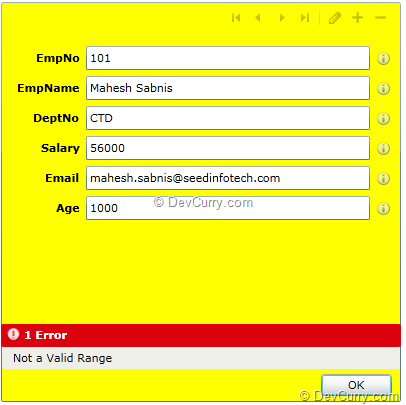
Likewise, you can validate all entries while performing Insert/Update operations.
Conclusion: DataAnnotation is a feature using which validation logic can be isolated from the UI. Typically this helps in the code-less development.
Tweet
No comments:
Post a Comment