1) DataGrid.
2) TextBox.
3) Image.
So let’s add a XML file to our WPF project and call it ‘Customers.xml’. Now add some dummy data as shown below –
<?xml version="1.0" encoding="utf-8" ?> <Customers> <Customer CustomerID="98886" Name="Pravinkumar R. D." City="Pune"> <OrderNo>100</OrderNo> <ProductName>Samsung Monitor</ProductName> <Picture>Images/samsungmonitor.jpg</Picture> <Price>$1200</Price> </Customer> <Customer CustomerID="98887" Name="Alisha C." City="Mumbai"> <OrderNo>200</OrderNo> <ProductName>Samsung LCD</ProductName> <Picture>Images/samsunglcd.jpg</Picture> <Price>$12400</Price> </Customer> <Customer CustomerID="98888" Name="Yash D." City="Delhi"> <OrderNo>300</OrderNo> <ProductName>Samsung iPAD</ProductName> <Picture>Images/SamsungTab.jpg</Picture> <Price>$120</Price> </Customer> <Customer CustomerID="98889" Name="Mahesh S." City="Mumbai"> <OrderNo>400</OrderNo> <ProductName>Samsung Laptop</ProductName> <Picture>Images/samsunglaptop.jpg</Picture> <Price>$1500</Price> </Customer> </Customers>
For this demonstration, I have added a folder called ‘Images’ in the WPF project, with a few sample images. Now let’s add a XML data source in our XAML code, which will fetch the data from our XML file ‘Customers.xml’ as shown below –
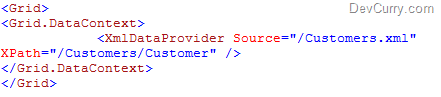
After this step, let’s design the main UI which will display all the customers in a Datagrid control. When we choose a specific customer by selecting a DataGrid row, it will show the detailed order for that customer. For this let’s add some XAML code as shown below –
<Grid.RowDefinitions> <RowDefinition Height="130*" /> <RowDefinition Height="181*" /> </Grid.RowDefinitions> <DataGrid x:Name="dataGrid1" Margin="8,8,8,0" AutoGenerateColumns="False" ItemsSource="{Binding}" Height="114" VerticalAlignment="Top" IsSynchronizedWithCurrentItem="True" Grid.Row="0"> <DataGrid.Columns> <DataGridTextColumn Binding="{Binding XPath=@CustomerID}" Header="CustomerID"/> <DataGridTextColumn Binding="{Binding XPath=@Name}" Header="Name"/> <DataGridTextColumn Binding="{Binding XPath=@City}" Header="City"/> </DataGrid.Columns> </DataGrid> <StackPanel Orientation="Vertical" Grid.Row="1"> <StackPanel Orientation="Horizontal"> <TextBlock Text="Order No."/> <TextBox Text="{Binding XPath=OrderNo}" Width="83" /> </StackPanel> <StackPanel Orientation="Horizontal"> <TextBlock Text="Product Name"/> <TextBox Text="{Binding XPath=ProductName}"/> </StackPanel> <StackPanel Orientation="Horizontal"> <TextBlock Text="Product Price"/> <TextBox Text="{Binding XPath=Price}"/> </StackPanel> <StackPanel Orientation="Horizontal"> <Image Source="{Binding XPath=Picture}" Height="100" Width="200"/> </StackPanel> </StackPanel>
If you observe, the DataGrid property ‘IsSynchronizedWithCurrentItem’ is set to true. This means, when we make a choice of the DataGrid row, the corresponding item will be shown below as a details view in the TextBox control. It will also show an image of the product in an Image control.
Now when you run the project and select any row of the DataGrid, the output will look similar to the following –
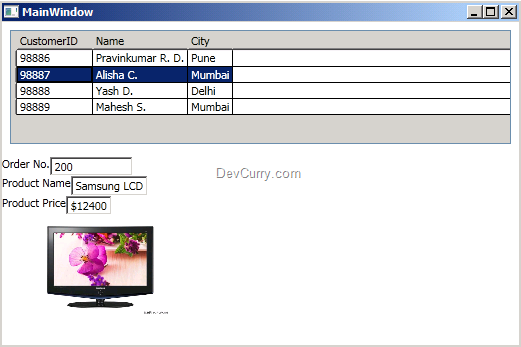
Tweet
2 comments:
Goody! Can the same be done for a Silverlight Grid too. I do not have a a machine with VS now so thought of asking you directly.
Hi Mufto,
You can not do the same in Silverlight. However, in Silverlight 4.0, there are few capabilities which you can use. Please go through this link -
http://mtaulty.com/CommunityServer/blogs/mike_taultys_blog/archive/2010/04/19/silverlight-and-xml-binding.aspx
Post a Comment