In this article, we will look at the <picture> element and how it helps in responsive design to render images of different sizes, based on the physical device accessing the content.
To display an image in our HTML pages, we have so far made use of the <img/> element with the source [src] attribute. Here’s an example:
With varying screen sizes and pixels, handling this image becomes a challenge. Let's create a small example for demonstrating this. I am using the free Visual Studio Community Edition although you can use any other web editor of your choice. Create an empty web application and add a HTML page with the name TestPicture.html in our project. Add the following code:
Now browse the page and see the output.

Now if you try resizing the browser, you will be able to display the same image under many different sizes. However you won’t be able to use different images for differing sizes, viewport height, width, or pixel density. Try browsing this page on mobile, tablets or desktop kind of devices and you will see what I mean.
A typical requirement could be what if we would like to provide responsive images to the user based on the physical screen size, users' screen pixel density, users' zoom level or screen orientation. And most importantly, what if we would like to display a different image with the different contents, depending on the rendered size of the image.
In these scenarios, you can make use of HTML5’s <picture/> element. Let's change the code of our HTML page as shown here -
Now let's browse the web page and resize the browser width. I am using Google Chrome for this demonstration. The output should appear as follows:
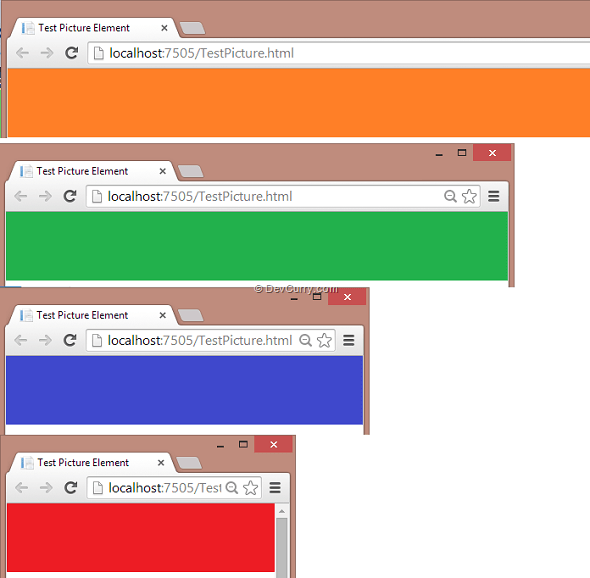
In the above figure, you will see the different images based on the size of the browser. We have used five different images in the <picture/> element. The picture element contains <source/> element. This source element contains a couple of attributes like -
· srcset - Image file path. It can also contain a comma delimited list of images with pixel density descriptor.
· media - Contains media query
It also contains <img/> element for a fallback scenario. In case the browser does not support <picture/> element, it will make use of the <img/> element. For example, if you try browsing the element in IE 11, you will see the following output:

Also, if you are using Visual Studio 2015 as your web editor, you will see a CSS class categorization for eg: we are using bootstrap.css into our page as well as our own CSS.
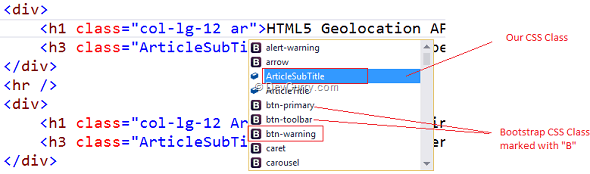
In case you want to know which browsers support the <picture/> element as of today, use the following link - http://caniuse.com/#search=picture
In this article, we saw how to provide responsive images in HTML5 using the <picture/> element.
To display an image in our HTML pages, we have so far made use of the <img/> element with the source [src] attribute. Here’s an example:
<img src="Images/default.png" alt="Default Logo" />
With varying screen sizes and pixels, handling this image becomes a challenge. Let's create a small example for demonstrating this. I am using the free Visual Studio Community Edition although you can use any other web editor of your choice. Create an empty web application and add a HTML page with the name TestPicture.html in our project. Add the following code:
<!DOCTYPE html> <html lang="en"> <head> <title>Test Picture Element</title> <link href="Content/bootstrap.min.css" rel="stylesheet" /> <link href="Content/MyCSS.css" rel="stylesheet" /> </head> <body> <img src="Images/default.png" alt="Default Logo" /> </body> </html>
Now browse the page and see the output.

Now if you try resizing the browser, you will be able to display the same image under many different sizes. However you won’t be able to use different images for differing sizes, viewport height, width, or pixel density. Try browsing this page on mobile, tablets or desktop kind of devices and you will see what I mean.
A typical requirement could be what if we would like to provide responsive images to the user based on the physical screen size, users' screen pixel density, users' zoom level or screen orientation. And most importantly, what if we would like to display a different image with the different contents, depending on the rendered size of the image.
In these scenarios, you can make use of HTML5’s <picture/> element. Let's change the code of our HTML page as shown here -
<!DOCTYPE html> <html lang="en"> <head> <title>Test Picture Element</title> <link href="Content/bootstrap.min.css" rel="stylesheet" /> <link href="Content/MyCSS.css" rel="stylesheet" /> </head> <body> <!--<img src="Images/default.png" alt="Default Logo" />--> <picture> <source media="(min-width: 1024px)" srcset="Images/default.png" /> <source media="(min-width: 650px)" srcset="Images/green.png" /> <source media="(min-width: 450px)" srcset="Images/blue.png" /> <source media="(min-width: 350px)" srcset="Images/red.png" /> <img src="Images/fallback.png" alt="Logo" style="height:90px;width:1024px" /> </picture> <div class="row"> <div> <h1 class="col-lg-12">The Absolutely Awesome jQuery Cookbook Released</h1> <h3 class="ArticleSubTitle">Saturday, December 13, 2014 Posted by Suprotim Agarwal</h3> </div> <hr /> <div> <h1 class="col-lg-12 ArticleTitle">HTML5 Geolocation API - Getting Started</h1> <h3 class="ArticleSubTitle">Thursday, November 20, 2014 Posted by Pravinkumar Dabade </h3> </div> <hr /> <div> <h1 class="col-lg-12 ArticleTitle">Tracing in ASP.NET Web API </h1> <h3 class="ArticleSubTitle">Thursday, October 23, 2014 Posted by Suprotim Agarwal </h3> </div> </div> </body> </html>
Now let's browse the web page and resize the browser width. I am using Google Chrome for this demonstration. The output should appear as follows:
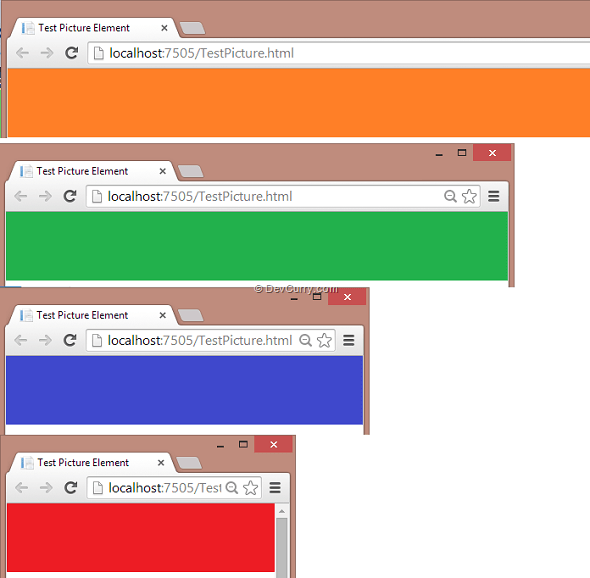
In the above figure, you will see the different images based on the size of the browser. We have used five different images in the <picture/> element. The picture element contains <source/> element. This source element contains a couple of attributes like -
· srcset - Image file path. It can also contain a comma delimited list of images with pixel density descriptor.
· media - Contains media query
It also contains <img/> element for a fallback scenario. In case the browser does not support <picture/> element, it will make use of the <img/> element. For example, if you try browsing the element in IE 11, you will see the following output:

Also, if you are using Visual Studio 2015 as your web editor, you will see a CSS class categorization for eg: we are using bootstrap.css into our page as well as our own CSS.
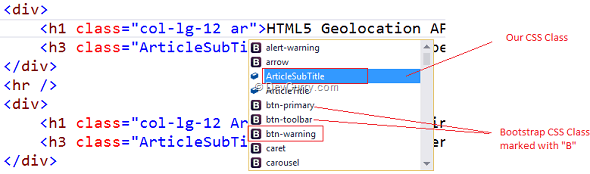
In case you want to know which browsers support the <picture/> element as of today, use the following link - http://caniuse.com/#search=picture
In this article, we saw how to provide responsive images in HTML5 using the <picture/> element.
Tweet
No comments:
Post a Comment