Geolocation is one of the first HTML5 features that has been embraced across all major browsers. In this article, we will look at the HTML5 Geolocation API to get/access a user’s Geolocation.
Using HTML5 Geolocation API, you can share your current location with trusted web sites or even use it to provide additional geo location features in your own website, like providing discounts to visitors of your city. Finding out a visitor’s current location can be done using various methods. For example –
We will try this with an example. I am using Visual Studio 2012 for this demonstration although you can use any web editor of your choice. We will first create an empty web application using Visual Studio as shown below –
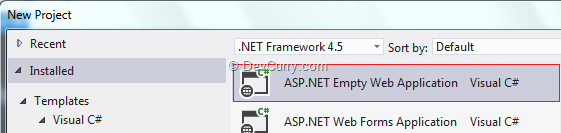
We will add support for jQuery and Modernizer in our project using NuGet package. Right click your project in Solution Explorer and click on “Manage NuGet Packages” and install jQuery and Modernizer in your project.
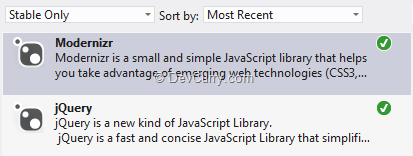
Now we will add a HTML page with the name MyLoc.html. Change the title to “HTML-5 GeoLocation API Test” and add the jQuery and Modernizer script reference into your HTML page. The Page should look like the following –
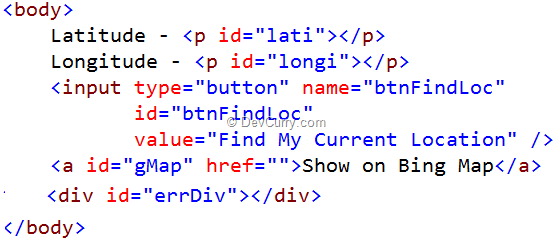
Let’s design the HTML page. Add the following code in the body tag –
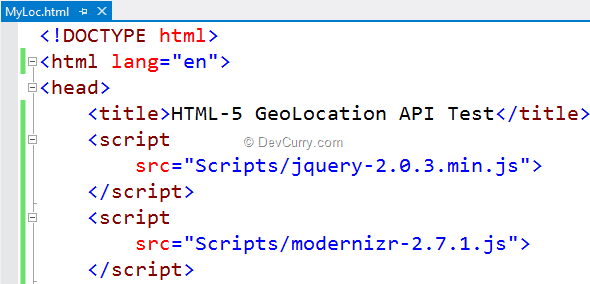
It’s time to check whether your browser supports the Geolocation API or not. For this we can make use of Modernizr library. We will also write some code for fetching the latitude and longitude using jQuery. Let’s write the following code before the body tag ends –
<script>
$(function () {
var error = $('#errDiv');
$('#btnFindLoc').click(function () {
if (Modernizr.geolocation) {
navigator.geolocation.getCurrentPosition(currentPosition, positionError);
}
else {
error.html("GeoLocation API of HTML 5 is not supported");
}
});
function currentPosition(currentPos) {
var coordinates = currentPos.coords;
$('#lati').text(coordinates.latitude);
$('#longi').text(coordinates.longitude);
var googleMap = $('#gMap');
googleMap.attr("href", "http://maps.google.com/maps?q=" + coordinates.latitude + "," + coordinates.longitude);
}
function positionError(errCode) {
if (errCode.code==0) {
error.html("Unknown Error - has occured");
}
else if (errCode.code==1) {
error.html("Permission Denied - By the user");
}
else if (errCode.code==2) {
error.html("Position/Location Unavailable");
}
else if (errCode.code == 3) {
error.html("Timeout");
}
}
});
</script>
In the above script, we are using the jQuery DOM Ready function, although its not really needed since the script is added just before the closing body tag. On the button click we are detecting the Geolocation support using Modernizr.geolocation. If your browser supports Geolocation, we call getCurrentPosition(success,fail) method. The currentPosition function fetches the Latitude and Longitude of the current location which in turn is passed to Google Maps.
When you call the getCurrentPosition method, the browser will not send your physical location to the web server. You will have to grant permission explicitly to do so. In IE 9.0+, you will see a permission dialog box as shown below –

Once you allow the information to be exposed, the code sets the “href” attribute value of anchor tag with Google Maps URL. When you click on the hyperlink, it will show you your location on Google Maps as shown below [Please note that this is my current location, in your case, Google maps will show you your location based on your coordinates]–
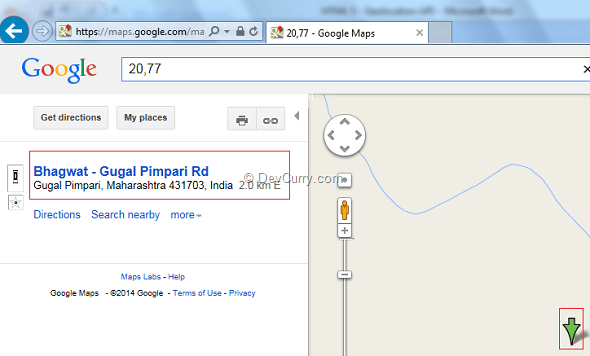
The positionError function deals with possible exceptions which may arise during the execution of our code. And that’s all there is to test the Geolocation API.
Summary – In this article, we have seen how to access/get the current position of the user using HTML 5 – Gelolocation API.
Using HTML5 Geolocation API, you can share your current location with trusted web sites or even use it to provide additional geo location features in your own website, like providing discounts to visitors of your city. Finding out a visitor’s current location can be done using various methods. For example –
- Find location using IP address
- Wireless network connection
- Using GPS etc
We will try this with an example. I am using Visual Studio 2012 for this demonstration although you can use any web editor of your choice. We will first create an empty web application using Visual Studio as shown below –
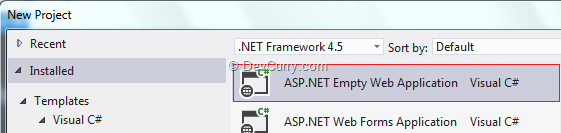
We will add support for jQuery and Modernizer in our project using NuGet package. Right click your project in Solution Explorer and click on “Manage NuGet Packages” and install jQuery and Modernizer in your project.
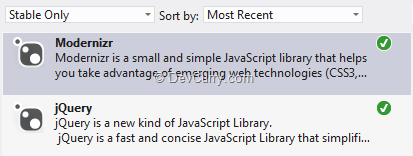
Now we will add a HTML page with the name MyLoc.html. Change the title to “HTML-5 GeoLocation API Test” and add the jQuery and Modernizer script reference into your HTML page. The Page should look like the following –
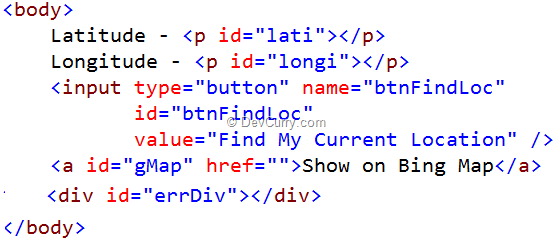
Let’s design the HTML page. Add the following code in the body tag –
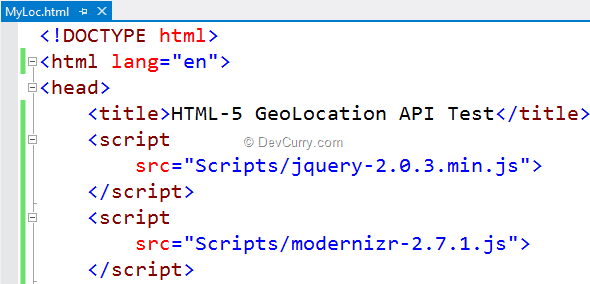
It’s time to check whether your browser supports the Geolocation API or not. For this we can make use of Modernizr library. We will also write some code for fetching the latitude and longitude using jQuery. Let’s write the following code before the body tag ends –
<script>
$(function () {
var error = $('#errDiv');
$('#btnFindLoc').click(function () {
if (Modernizr.geolocation) {
navigator.geolocation.getCurrentPosition(currentPosition, positionError);
}
else {
error.html("GeoLocation API of HTML 5 is not supported");
}
});
function currentPosition(currentPos) {
var coordinates = currentPos.coords;
$('#lati').text(coordinates.latitude);
$('#longi').text(coordinates.longitude);
var googleMap = $('#gMap');
googleMap.attr("href", "http://maps.google.com/maps?q=" + coordinates.latitude + "," + coordinates.longitude);
}
function positionError(errCode) {
if (errCode.code==0) {
error.html("Unknown Error - has occured");
}
else if (errCode.code==1) {
error.html("Permission Denied - By the user");
}
else if (errCode.code==2) {
error.html("Position/Location Unavailable");
}
else if (errCode.code == 3) {
error.html("Timeout");
}
}
});
</script>
In the above script, we are using the jQuery DOM Ready function, although its not really needed since the script is added just before the closing body tag. On the button click we are detecting the Geolocation support using Modernizr.geolocation. If your browser supports Geolocation, we call getCurrentPosition(success,fail) method. The currentPosition function fetches the Latitude and Longitude of the current location which in turn is passed to Google Maps.
When you call the getCurrentPosition method, the browser will not send your physical location to the web server. You will have to grant permission explicitly to do so. In IE 9.0+, you will see a permission dialog box as shown below –

Once you allow the information to be exposed, the code sets the “href” attribute value of anchor tag with Google Maps URL. When you click on the hyperlink, it will show you your location on Google Maps as shown below [Please note that this is my current location, in your case, Google maps will show you your location based on your coordinates]–
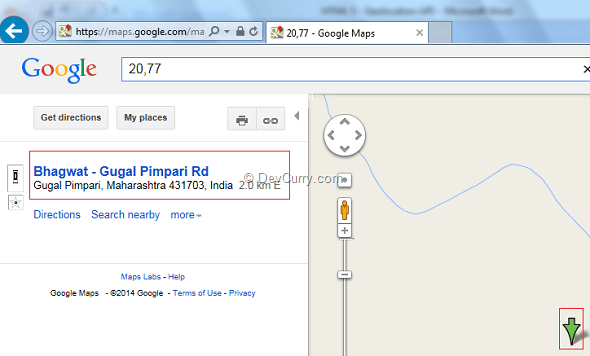
The positionError function deals with possible exceptions which may arise during the execution of our code. And that’s all there is to test the Geolocation API.
Summary – In this article, we have seen how to access/get the current position of the user using HTML 5 – Gelolocation API.
Tweet
No comments:
Post a Comment