To manage data of web applications, Backbone provides a Model creation strategy. The model allows us to define properties to contain data and business logic. The Collection declaration allows to store collection of the data, which is later used by the view.
In Backbone, the View (UI) is generally rendered using HTML Templates, and elements declared in this Template are accessible to View, but it might be possible that the HTML page (or MVC view or .aspx) already contains HTML elements and you need to handle the DOM events for these elements. The question then is how to handle them?
In the following implementation we will see that the ‘click’ event for the html button element is handled inside the View object. The method which gets executed for the click event is used to add the model object into the collection and then the collection is rendered using HTML Table.
Step 1: Open Visual Studio 2012/2013 and create a new ASP.NET Empty web application. In this project, add Backbone and jQuery libraries using NuGet Package.
Step 2: In this project, add a new folder and name it as ‘Backcone_Custom_Scripts’. In this folder add a new JavaScript file and name it as Model.js. Define the following Model and Collection objects in Model.js
var Employee = Backbone.Model.extend({
defaults: {
EmpNo: 0,
EmpName: "",
DeptName: "",
Designation:"",
Salary:0.0
}
});
//Declaring Employee Collection
var EmployeesCollection = Backbone.Collection.extend({
model: Employee
});
Step 3: Add a new HTML page in the project and name it as ‘H4_Adding_Data_To_Collection_DOM_Events.html’. Add the following script reference in it:
<script src="Scripts/jquery-2.0.3.min.js"></script>
<script src="Scripts/underscore.min.js"></script>
<script src="Scripts/backbone.min.js"></script>
<script src="Backcone_Custom_Scripts/Models.js"></script>
Step 4: In the <body> tag of the page, add a <table> and in the table add Textboxes and Buttons. Add a <div> tag below the table to render collection:
<table style="width:100%;" id="tblinput">
<tr>
<td>EmpNo:</td>
<td>
<input type="text" id="txteno" placeholder="EmpNo is Numeric" />
</td>
</tr>
<tr>
<td>EmpName:</td>
<td>
<input type="text" id="txtename" placeholder="EmpName is Text" />
</td>
</tr>
<tr>
<td>DeptName:</td>
<td>
<input type="text" id="txtdname" placeholder="DeptName is Text" />
</td>
</tr>
<tr>
<td>Designation:</td>
<td>
<input type="text" id="txtdesig" placeholder="Designation is Text" />
</td>
</tr>
<tr>
<td>Salary:</td>
<td>
<input type="text" id="txtsal" placeholder="Salary is Numeric" />
</td>
</tr>
<tr>
<td>
<button id="btnadd">Add</button>
</td>
<td>
<button id="btnclear">Clear</button>
</td>
<td>
<input type="checkbox" id="ckbksort" value="Sory by Name" />
</td>
</tr>
</table>
<div id="dvcontainer"></div>
Step 5: Add the script in the <body> tag below the <div> with id ‘dvcontainer’, This will define the View object as shown here:
var EmployeeRecordsView = Backbone.View.extend({
//The body element in which all elements are displayed
el: 'body',
//Events for the elements on the page.
events:{
'click button#btnadd': 'addData',
'click button#btnclear': 'clearInput'
},
//Render function to display collection collection in the Table
render: function () {
var dvcontainer = $(this.el).find('#dvcontainer');
var viewHtml = '<table border="1">';
viewHtml += "<tr><td>EmpNo</td><td>EmpName</td><td>DeptName</td><td>Designation</td> <td>Salary</td></tr>";
//Iterate through the collection
_.each(this.collection.models, function (m, i, l) {
var empRecHtml = '<tr><td>' + m.get('EmpNo') + '</td><td>' + m.get('EmpName') + '</td><td>' + m.get('DeptName') + '</td><td>' + m.get('Designation') + '</td><td>' + m.get('Salary') + '</td></tr>';
viewHtml += empRecHtml;
});
viewHtml += '</table>';
dvcontainer.html(viewHtml);
},
//Function executed on the button clieck
addData: function () {
var newEmp = new Employee();
newEmp.set('EmpNo', $("#txteno").val());
newEmp.set('EmpName', $("#txtename").val());
newEmp.set('DeptName', $("#txtdname").val());
newEmp.set('Designation', $("#txtdesig").val());
newEmp.set('Salary', $("#txtsal").val());
EmpCollection.add(newEmp);
},
//Function to clear all textboxes.
clearInput: function () {
//Clear all Textboxes
$("#tblinput input").val('');
}
});
The above code has some important features:
- The ‘el’ element is defined as ‘body’ to display all elements in it.
- ‘events’ defines ‘click’ event for the button on the Page. These buttons are found using jQuery selector using #‘. Method is assigned to each event i.e. addData and clearInput.
- The ‘render’ function defines the logic for finding the <div> having id as ‘dvContainer’. Once it is found, then the HTML table is generated by iterating through the collection passed to the view. (The code is provided in future steps).
- The ‘addData’ method reads values entered in the Textboxes and using the ‘set’ method of the Backbone model, the value entered in the Textbox is passed to an individual property of the Employee Model object. The model is then added into the EmployeeCollection using ’add()’ method of the Backbone collection.
- The ‘clearInput’ method clears all Textboxes.
//The Collection Object
var EmpCollection = new EmployeesCollection();
//The View Object and Pass Collection to it
var EmpsView = new EmployeeRecordsView({
collection:EmpCollection
});
Step 7: Since we need to add data entered in Textboxes to the collection and render the data from collection in a Table, subscribe to the ‘add’ event of the collection and call ‘render’ method of the View in it. So when the collection is changed, a new row will be added in the table.
//Subscribe to the 'add' event of the collection
//When Collection changed
//Render the Updated Table View
EmpCollection.on('add', function () {
EmpsVeiw.render();
});
Step 8: View the page in browser
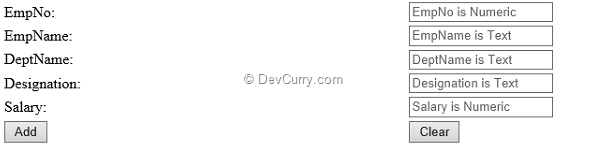
Enter some data in each Textbox and click on ‘Add’. The data will get added in the Table as shown below:
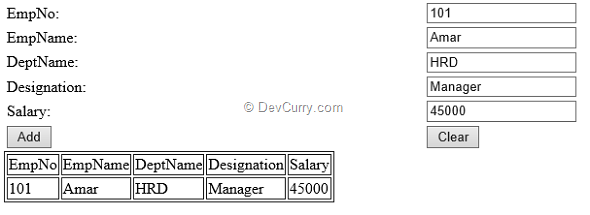
Click on the ‘Clear’ button to clear all Textboxes. Enter new record values in Textboxes and click on Add button and a new row will be added in the table:
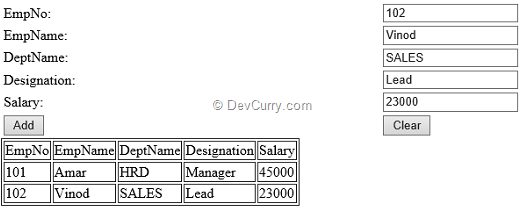
Conclusion: The Backbone library allows you to develop responsive web applications with clear separation of Model, View. Events associated with Collection helps to notify the UI elements about Updates and accordingly to display refreshed data.
Tweet
No comments:
Post a Comment