This article discusses when should one think about an Offline Web Application. What is an HTML5 offline application and to create one.
When should think about creating an Offline web application?
There are many situations where creating an Offline Web Applications makes sense. Some of them are:
What is an HTML 5 Offline Application?
When you think about web applications, the common scenario is you render the web pages dynamically based on some actions that you perform on your web page. For example, click a link button which will render another web page from the server. But all this is possible only when you are online; which means you have network connectivity. But what if you don’t have network connectivity. Obviously, you will not be able to render any web page from the server unless it is cached.
This is where your HTML offline application will come into the picture. You can download your required pages when you are online. If network connectivity goes off, HTML 5 offline application will automatically serves the offline web pages which you have downloaded when you were online.
To create an offline HTML 5 web application, you will have to create a “Manifest” file which contains the URLs of the resources which you want to download. The resources could be –
To implement this demonstration, I am using Visual Studio 2013 although you can use a previous edition too. You can also use any other Web Editor of your choice. Now let’s start by creating an ASP.NET Web application (not a requirement). Open Visual Studio and click on New Project. Choose ASP.NET Web Application. Name it “HTML5OfflineApplication”. In the dialog box, choose a Web Form with Empty template.
Once you are ready with the application, we will not add few contents in our application as described below –
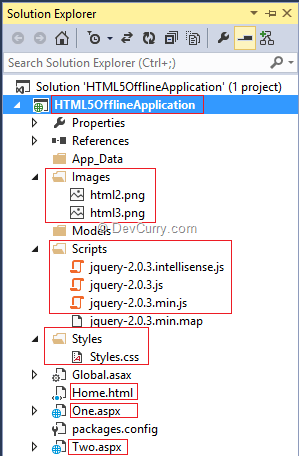
Now let’s design One.aspx and Two.aspx. Add a Heading tag <h1> and Image in both the pages respectively as shown below –
<h1>Web Page One</h1>
<asp:Image ImageUrl="~/Images/html2.png" runat="server"/>
<h1>Web Page Two</h1>
<asp:Image ImageUrl="~/Images/html3.png" runat="server"/>
Change the image names to your images which you have added in your Images folder.
Now let’s add some Styles in our Styles.css file as shown below –
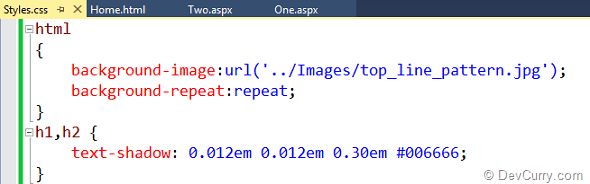
Let’s design our Home page as follow –
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>HTML 5 Offline Web Application</title>
</head>
<body>
<h1>HTML 5 Offline Application Example</h1>
<h2>WelCome To HTML 5 Offline Application</h2>
<h3>Application Status - : <span id="networkStatus"></span></h3>
<a href="One.aspx">Go To Page 1</a><br />
<a href="Two.aspx">Go To Page 2</a></body>
</html>
The above Home page is a simple web page which has <h1>, <h2>, <h3> and <a> tags. Drag and drop the Styles.css file in our <head> tag of Home.html page and run the page. You should see the following:
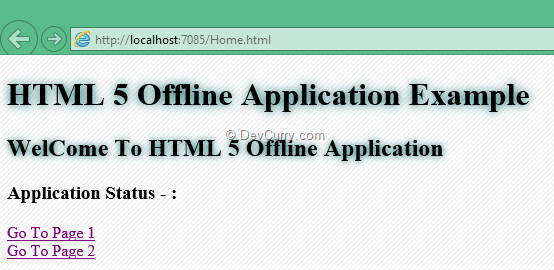
Our next step is to identify whether the application is online or offline. For this, we will make use of jQuery’s document.ready() function and will check after a certain interval whether you are connected or are offline. Drag and drop jQuery file in our head tag and write the following code in your Document Ready function –

Editor’s note: You can also use the online and offline events of the browser like this:
$(document).on('offline online', function (event) {
$("#networkStatus").html('You are ' + event.type + '!');
});
Now we have a network status displayed on our web page, we will prepare our Manifest to offline resources. The cache manifest file is a text file that lists the resources the browser should cache for offline access. Let’s add a text file with the name devcurry.manifest in our web application. The manifest looks like below.
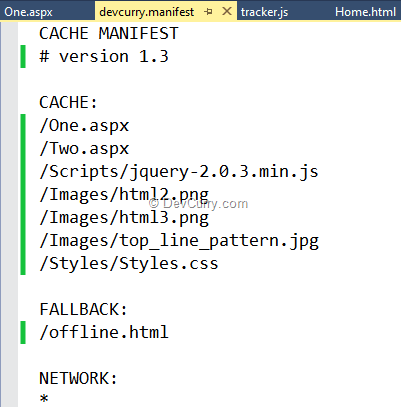
In the above manifest, we are first deciding which resources (.ASPX, .JS, .PNG, .JPG, .CSS) should be offline with the version number of Manifest. After that we are setting up a Fallback. When you do not download the resource or the resource doesn’t get download, the Fallback page will come into the picture.
The last section is Network. This section tells that in case whatever resource you are looking for is not available in the cache, it can be downloaded from the given address. This address can be specific as well, like your web address.
Now we ready with the manifest, let’s configure the manifest in our Home page as shown below –
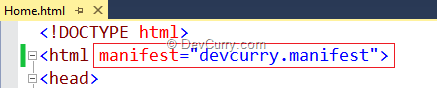
Let’s try some events which will occur when you are caching the application for offline viewing. For this we will add an unordered list in a div tag on our Home.html page. The code looks like the following –
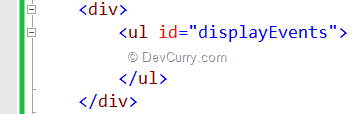
Now we will program against the events of application cache. Add the following script before the </body> tag –
<script>
function Track(output) {
$("#displayEvents").append("<li>" + output + "</li>");
}
$(function () {
if (window.applicationCache) {
window.applicationCache.onchecking = function (e) {
Track("Currently we are checking the cache");
}
window.applicationCache.oncached = function (e) {
Track("The contents are Cached");
}
window.applicationCache.onnoupdate = function (e) {
Track("There are no Updates");
}
window.applicationCache.ondownloading = function (e) {
Track("Downloading the contents for site!!");
}
window.applicationCache.onerror = function (e) {
alert(window.applicationCache.status);
Track("Something Went wrong" + e.message);
}
Track("Just Loaded the Window");
window.applicationCache.onupdateready = function (e) {
applicationCache.swapCache();
Track("Cache Updated Successfully");
}
}
})
</script>
This script will check the various states of the application cache and will get executed as per the changes. Now when you run your web page which is Home.html, you will see an output like below –
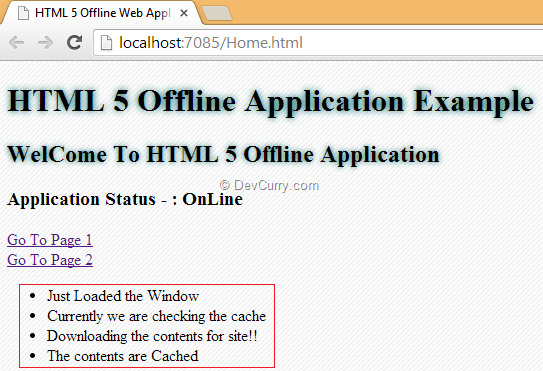
Now change the version of manifest from 1.3 to 1.4 and rerun the page and check what happens. You should see the following output -
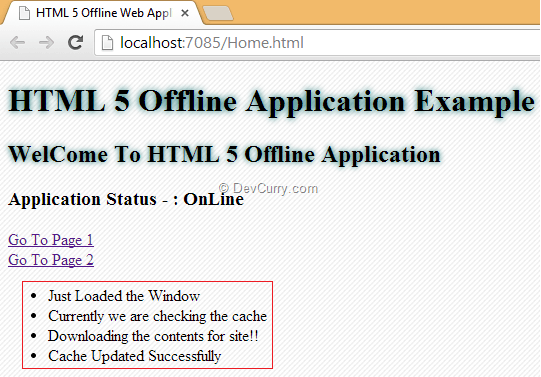
Notice the last event message is Cache Updated Successfully. This is because we are online and we made changes to the manifest file. Now take the application offline and click on the links and your application will still work as is with the status “offline” as shown below –
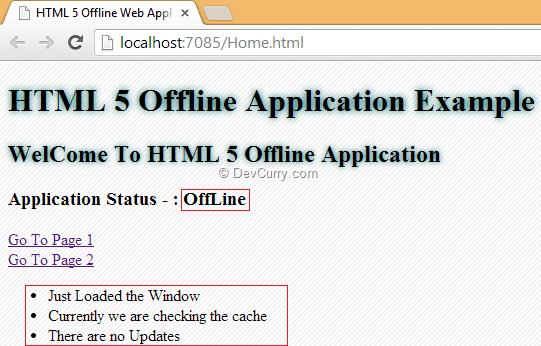
Note – the cache size of the data differs browser to browser. Typically it is 5 MB. Also if you do not see any of this, probably your browser does not support HTML5.
Conclusion – In this article, we have seen how to make an offline Web Application using the HTML5 application cache.
When should think about creating an Offline web application?
There are many situations where creating an Offline Web Applications makes sense. Some of them are:
- You may want to work with your application even when you are outside your company/organization’s network.
- You may want to view documents/web pages when you are offline with no network connectivity.
- You may have data entry application where you are storing data offline and once the network is available, you want to synchronize the data back to your main application.
- In case if you are working with media applications, you may want some offline media pages which allows you to see videos offline etc.
What is an HTML 5 Offline Application?
When you think about web applications, the common scenario is you render the web pages dynamically based on some actions that you perform on your web page. For example, click a link button which will render another web page from the server. But all this is possible only when you are online; which means you have network connectivity. But what if you don’t have network connectivity. Obviously, you will not be able to render any web page from the server unless it is cached.
This is where your HTML offline application will come into the picture. You can download your required pages when you are online. If network connectivity goes off, HTML 5 offline application will automatically serves the offline web pages which you have downloaded when you were online.
To create an offline HTML 5 web application, you will have to create a “Manifest” file which contains the URLs of the resources which you want to download. The resources could be –
- Web pages
- CSS
- JavaScript
- Images
- Etc
To implement this demonstration, I am using Visual Studio 2013 although you can use a previous edition too. You can also use any other Web Editor of your choice. Now let’s start by creating an ASP.NET Web application (not a requirement). Open Visual Studio and click on New Project. Choose ASP.NET Web Application. Name it “HTML5OfflineApplication”. In the dialog box, choose a Web Form with Empty template.
Once you are ready with the application, we will not add few contents in our application as described below –
- Two .aspx pages with the name One.aspx and Two.aspx.
- Add a folder Styles and add a Styles.css style sheet in this folder.
- Add a folder Images and add any two images in this folder.
- Add a HTML page with the name Home.html.
- Add a Scripts folder and add jQuery files using NuGet packages.
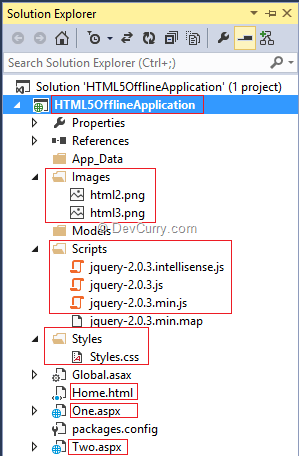
Now let’s design One.aspx and Two.aspx. Add a Heading tag <h1> and Image in both the pages respectively as shown below –
<h1>Web Page One</h1>
<asp:Image ImageUrl="~/Images/html2.png" runat="server"/>
<h1>Web Page Two</h1>
<asp:Image ImageUrl="~/Images/html3.png" runat="server"/>
Change the image names to your images which you have added in your Images folder.
Now let’s add some Styles in our Styles.css file as shown below –
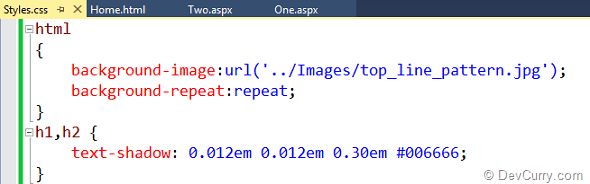
Let’s design our Home page as follow –
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>HTML 5 Offline Web Application</title>
</head>
<body>
<h1>HTML 5 Offline Application Example</h1>
<h2>WelCome To HTML 5 Offline Application</h2>
<h3>Application Status - : <span id="networkStatus"></span></h3>
<a href="One.aspx">Go To Page 1</a><br />
<a href="Two.aspx">Go To Page 2</a></body>
</html>
The above Home page is a simple web page which has <h1>, <h2>, <h3> and <a> tags. Drag and drop the Styles.css file in our <head> tag of Home.html page and run the page. You should see the following:
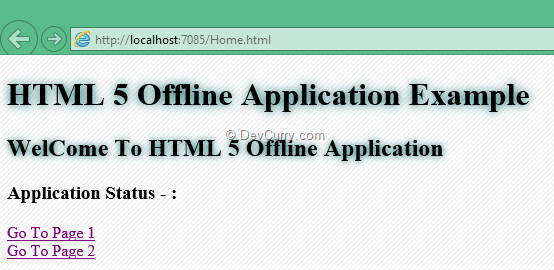
Our next step is to identify whether the application is online or offline. For this, we will make use of jQuery’s document.ready() function and will check after a certain interval whether you are connected or are offline. Drag and drop jQuery file in our head tag and write the following code in your Document Ready function –

Editor’s note: You can also use the online and offline events of the browser like this:
$(document).on('offline online', function (event) {
$("#networkStatus").html('You are ' + event.type + '!');
});
Now we have a network status displayed on our web page, we will prepare our Manifest to offline resources. The cache manifest file is a text file that lists the resources the browser should cache for offline access. Let’s add a text file with the name devcurry.manifest in our web application. The manifest looks like below.
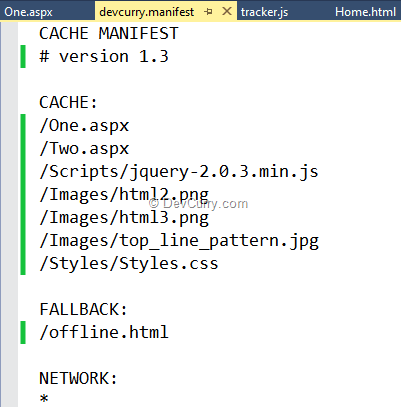
In the above manifest, we are first deciding which resources (.ASPX, .JS, .PNG, .JPG, .CSS) should be offline with the version number of Manifest. After that we are setting up a Fallback. When you do not download the resource or the resource doesn’t get download, the Fallback page will come into the picture.
The last section is Network. This section tells that in case whatever resource you are looking for is not available in the cache, it can be downloaded from the given address. This address can be specific as well, like your web address.
Now we ready with the manifest, let’s configure the manifest in our Home page as shown below –
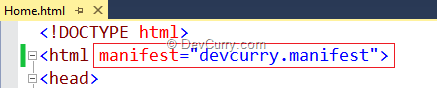
Let’s try some events which will occur when you are caching the application for offline viewing. For this we will add an unordered list in a div tag on our Home.html page. The code looks like the following –
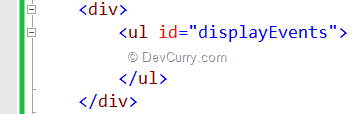
Now we will program against the events of application cache. Add the following script before the </body> tag –
<script>
function Track(output) {
$("#displayEvents").append("<li>" + output + "</li>");
}
$(function () {
if (window.applicationCache) {
window.applicationCache.onchecking = function (e) {
Track("Currently we are checking the cache");
}
window.applicationCache.oncached = function (e) {
Track("The contents are Cached");
}
window.applicationCache.onnoupdate = function (e) {
Track("There are no Updates");
}
window.applicationCache.ondownloading = function (e) {
Track("Downloading the contents for site!!");
}
window.applicationCache.onerror = function (e) {
alert(window.applicationCache.status);
Track("Something Went wrong" + e.message);
}
Track("Just Loaded the Window");
window.applicationCache.onupdateready = function (e) {
applicationCache.swapCache();
Track("Cache Updated Successfully");
}
}
})
</script>
This script will check the various states of the application cache and will get executed as per the changes. Now when you run your web page which is Home.html, you will see an output like below –
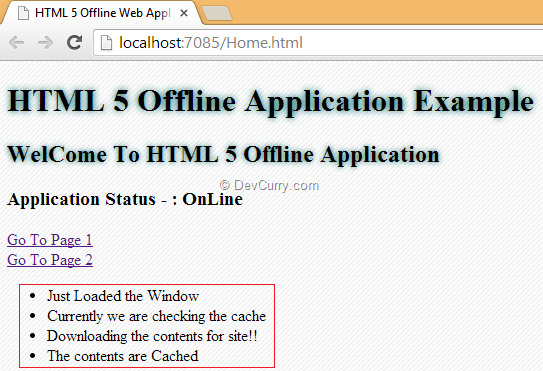
Now change the version of manifest from 1.3 to 1.4 and rerun the page and check what happens. You should see the following output -
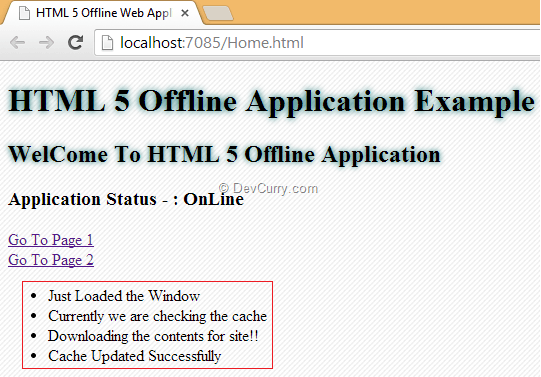
Notice the last event message is Cache Updated Successfully. This is because we are online and we made changes to the manifest file. Now take the application offline and click on the links and your application will still work as is with the status “offline” as shown below –
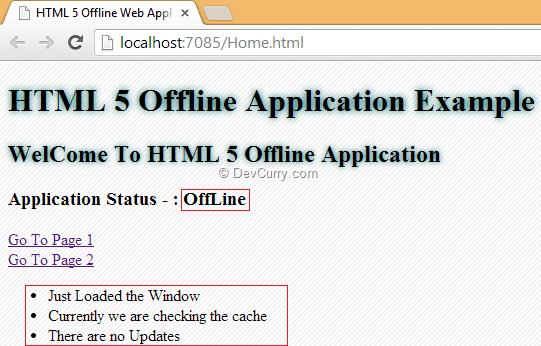
Note – the cache size of the data differs browser to browser. Typically it is 5 MB. Also if you do not see any of this, probably your browser does not support HTML5.
Conclusion – In this article, we have seen how to make an offline Web Application using the HTML5 application cache.
Tweet
1 comment:
Great Job!
I am working on a proposal and the RFP needs are to have a web application with offline capabilities. The Offline capabilities are required for an extensive data entry process where there are about 5 Chapters, tons of Grids and hundreds of Questions. This data entry process needs to be implemented through a web interface ensuring that the users from the countries who have unreliable internet connectivity can work offline for most part of the data entry work (may be for days and weeks) and once they are ready with the data entered, then the application should be able to sync it back to the main database....
Do you think HTML5 is mature enough to handle such complex and high volume offline data??
thanks and regards
Sumeet
Post a Comment