Getting Started – Installing MvcScaffolder
Step 1: We start off by creating a new MVC 4 Application in Visual Studio. We use the Basic Application template for now.Step 2: Install the MvcScaffolder using Nuget Package Manager
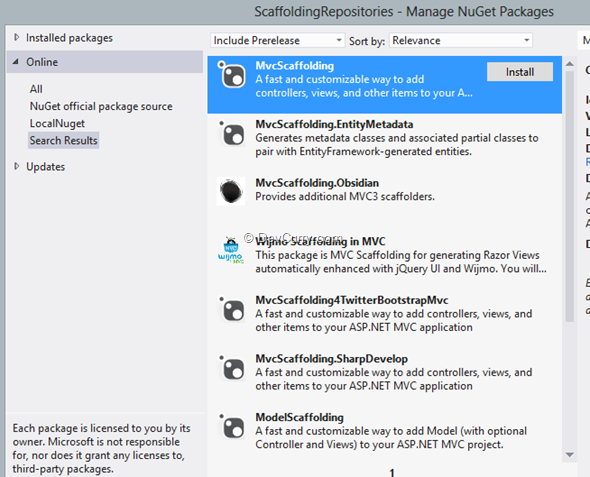
This will install the T4Scaffolding dependency as well.
Adding a Model entity and generating the CRUD Screens
Now that we have the MvcScaffolding package installed we are ready to setup our Model and then run the scaffolder on it.Step 3: Add the following Contact class in the Model folder and build the application.
public class Contact
{
public int Id { get; set; }
public string Name { get; set; }
public string Address { get; set; }
public string Email { get; set; }
public string Phone { get; set; }
}
Step 4: Right Click on the Controller folder and select Add Controller. It brings up the standard Add Controller dialog but if you look at the DropDown, it has two additional options thanks to MvcScaffolding.
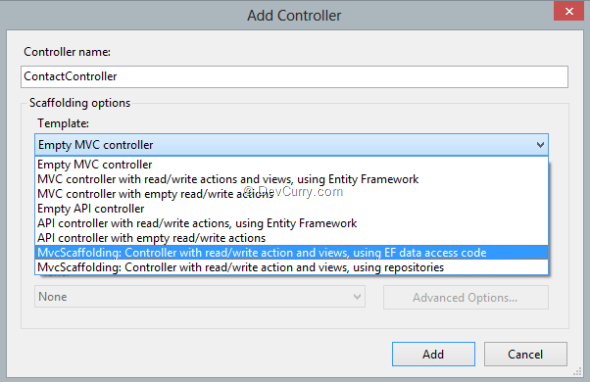
Step 5: Now setup the Add Controller dialog as follows to generate CRUD screens for Contact model object.
- Controller Name: ContactController
- Template as MvcScaffolding: Controller with read/write action and views, using repositories.
- Model Class: Contact (ScaffoldingRepositories.Model)
- Data Context Class: ScaffoldingRepositories.Models.ScaffoldingRepositoriesContext
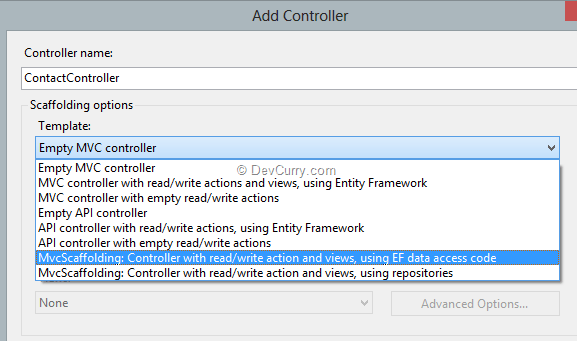
Click on Add to complete the scaffolding process.
Step 6: On completion of the Scaffolding process you will see the following files added to your solution
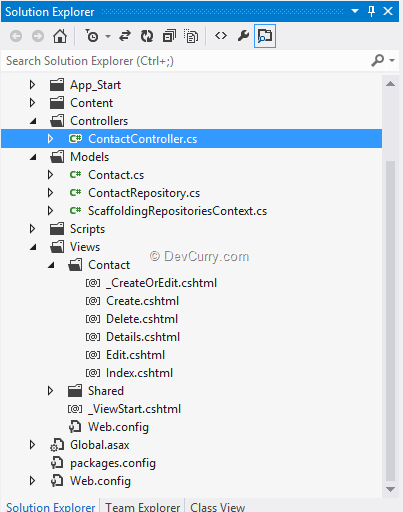
Controller – ContactController.cs
Models –
a. ContactRespository.cs: This has the Interface and the Implementation for persisting Contact Data. The implementation uses the next generated class i.e. ScaffoldingRepositoriesContext
b. ScaffoldingRepositoriesContext.cs: Contains the EF DbContext implementation used for persisting the data to LocalDb.
Views – Views has the standard CRUD views generated. One thing to note here is that there is a partial view called _CreateOrEdit.cshtml. This view as we will see shortly, is common to both Create and Edit screens and hence separated out into its own partial.
Applying Script References
The generated views have one minor gotcha, as in the script references for jQuery Validation are added at the top of the Create and Edit views.Our jQuery references are added at the bottom of the _Layout.cshtml. As a result of this the jQuery Validation scripts fail when trying to load ‘before’ the jQuery script itself.
The solution is simple.
Move the jQuery Validation references to the bottom of the page inside a Script section as follows
@section scripts{
<script src="@Url.Content("~/Scripts/jquery.validate.min.js")" type="text/javascript"></script>
<script src="@Url.Content("~/Scripts/jquery.validate.unobtrusive.min.js")" type="text/javascript"></script>
}
The Script section is important because in the _Layout.cshtml we have the following code
<body>
@RenderBody()
@Scripts.Render("~/bundles/jquery")
@RenderSection("scripts", required: false)
</body>
</html
What this means is, Razor view engine will add reference to the jQuery bundle to the Scripts section and then try to render the Scripts section if present in any other child views. The contents of the Scripts section in child view will be after the jQuery bundle has been rendered.
With the Script references fixed, we are ready to run the application.
Running the Application
When we run the application, now we should be able to save data to the database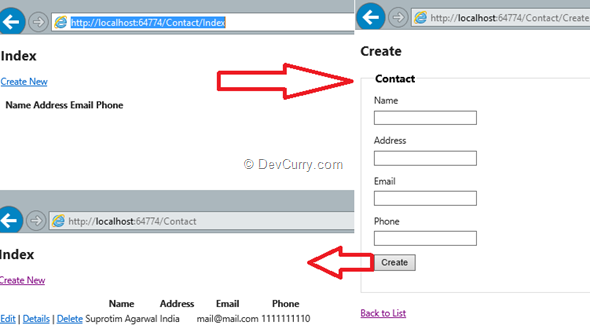
Neat! So our application is up and running as if it were built using the default MVC Scaffold tool. Time to dig in and see what’s different with the MvcScaffolding extension.
Digging deeper into generated code
Now that everything is working fine, let’s pop the hood and see how things are running. First up the repository.The Repository
The ContactRepository.cs has the Interface and the implementation for Contact Repository.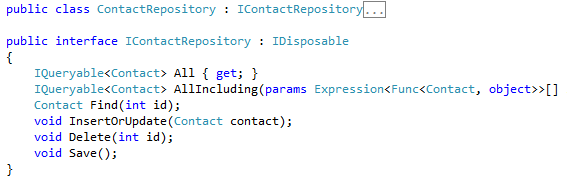
Note that the IContactRepository inherits from IDisposable. The implementation uses an instance of the ScaffoldingRepositoriesContext object that uses EF to persist data.
There is an interesting Repository method called AllIncluding and as we can see in the code below, it can accept a parameter consisting of an array of Model properties. This way we can limit the amount of data loaded if required. It can also be used for excluding Navigation properties etc.
The InsertOrUpdate method checks if the Id property has the default value, if it does, it saves it as a new entity. If the Id already has a non-default value, attempt to Update the entity.
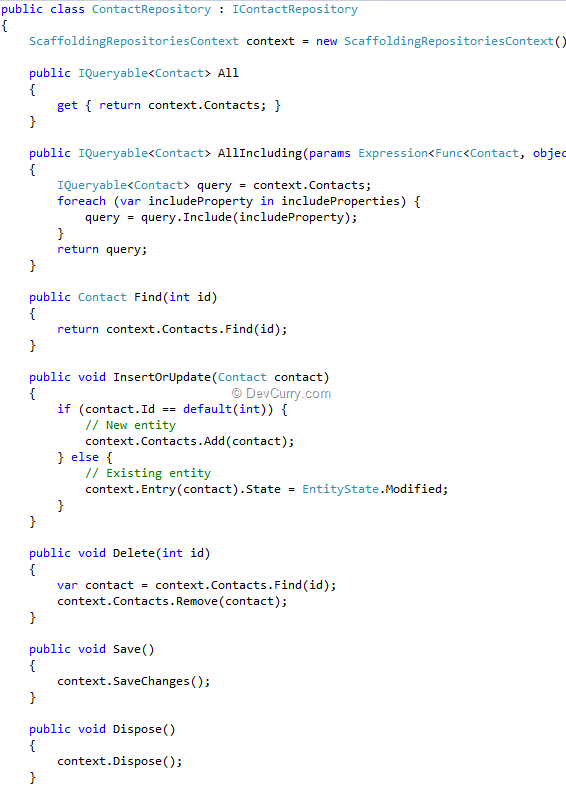
The ContactController
The ContactController is also generated a little differently, as in, instead of an instance of DbContext, we have a direct assignment of an Interface to its concrete class hardcoded in the constructor.private readonly IContactRepository contactRepository;
// If you are using Dependency Injection, you can delete the following constructor
public ContactController() : this(new ContactRepository())
{
}
To resolve this, we have to introduce an IoC Container to inject the dependency instance into the controller via the constructor. At this point, we can go ahead and integrate our favorite IoC container/DI framework as we want.
Other MvcScaffolding features
The MvcScaffolding package also has the ability to let you create your own Scaffolds. It creates a bootstrap set of files when you use the following command at the Package Manager ConsolePM> Scaffold CustomScaffolder MyScaffolder
What this does is, it adds T4 templates required for Scaffolding into a CodeTemplates folder. All T4 files are in a folder by the name of the scaffolder you created (MyScaffolder).
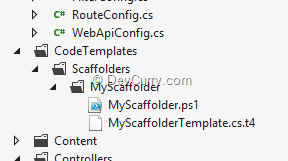
Now you can update the t4 template to generate a particular type of file and then run the Scaffolding as
PM> Scaffold MyScaffolder
This will generate the file ExampleOutput.cs as follows
namespace ScaffoldingRepositories
{
public class ExampleOutput
{
// Example model value from scaffolder script: Hello, world!
}
}
Essentially the Scaffolding plugin is not only helping generate CRUD screens, but if you have repetitive code that you want to automate, you can very well use the Custom Scaffolder feature to make life easier for yourself.
Conclusion
MvcScaffolding is a nifty extension that adds additional scaffolding capabilities to the default MVC tooling that comes out of the box.Caveat is that it’s not an officially supported Microsoft plugin. It was developed by Steve Sanderson and Scott Hanselman and you can find more information on Steve’s blog at http://blog.stevensanderson.com/?s=scaffolding
Download the entire source code of this article (Github)
Tweet
2 comments:
this no longer works.
vs 2013 + update 2 + mvcscaffolding
How do we get the "MvcScaffolding Controller with read/write actions and view, using repositories"?
I'm using VS 2015, MvcScaffolding 1.0.9, Mvc 5.2.3, but the Add Controller Dialog does not show that "repositories" option
Post a Comment