HTTP Services can be used by a broad range of clients including browsers and mobile devices. By using REST [Representational State Transfer] we can build loosely coupled services.
When we think about exposing data on the web, we always talk about four common operations which we use in our day-to-day life –
1. Create
2. Retrieve/Read
3. Update
4. Delete
We call these operations as CRUD operations. HTTP provides 4 basic verbs which we can map to our CRUD operations as described below –
1. POST – CREATE
2. GET – RETRIVE
3. PUT – UPDATE
4. DELETE – DELETE
By exposing your services over plain HTTP, if you have any application that can connect to the web, they can consume your data. In Web API, when the data is pulled or pushed by using HTTP, the data is always serialized as JSON (JavaScript Object Notation) or XML.
CREATE TABLE Customers
(
CustomerID VARCHAR(10) PRIMARY KEY,
ContactName VARCHAR(50),
City VARCHAR(50)
)
Update the ‘SampleDBEntities’ connection string to point to the correct DB Server and Instance.
Insert some dummy data that can be fetched. Once your table is ready, let’s add a new item in our Web Application. Right click the Web Application and add a new item which is “ADO.NET Entity Data Model” as shown below –
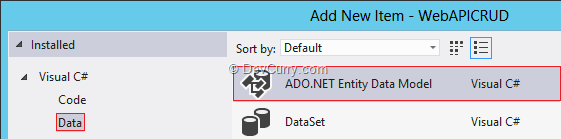
Now let’s follow the wizard steps. First Select “Generate from database”. Select connection string of your database. If the connection string is not available, create one and click on next button. Now in this step, Select Customers table from the Tables section and click on Finish button.
Now it’s time to implement our logic which will perform CRUD operations using ASP.NET Web API. When you are working with ASP.NET Web API, you will have to – Add a Web API Controller. Right click the ASP.NET Web Application and add a new Item. Select “Web API Controller Class”. Name it as “CustomersController” as shown below –
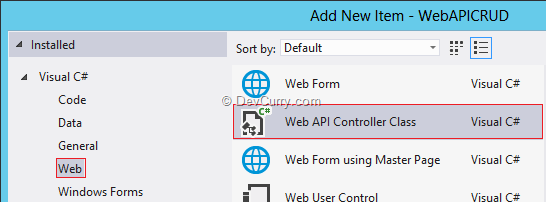
If you see, our “CustomersController” class is derived from ApiController class which belongs to System.Web.Http namespace as shown below –

This controller by default gives you the methods for your CRUD operations as described below –
1. Get () – Retrieve the data.
2. Get (int id) – Retrieve the data based on ID.
3. Post – Insert data.
4. Put – Update data.
5. Delete – Delete data.
Now let’s add a new class with the name “CustomersRepository” which will talk to our ADO.NET Entity framework which will then help our control to pull or push the data.
Once your class is ready, let’s add the following code to perform CRUD operations using our Entity Framework –
public class CustomersRepository
{
private static List<Customer> m_customersData;
public static List<Customer> GetAllCustomers()
{
SampleDBEntities dataContext=new SampleDBEntities();
var query = from customer in dataContext.Customers
select customer;
return query.ToList();
}
public static Customer GetCustomer(string customerID)
{
SampleDBEntities dataContext=new SampleDBEntities();
var query = (from customer in dataContext.Customers
select customer).SingleOrDefault();
return query;
}
public static void InsertCustomer(Customer newCustomer)
{
SampleDBEntities dataContext = new SampleDBEntities();
dataContext.Customers.Add(newCustomer);
dataContext.SaveChanges();
}
public static void UpdateCustomer(Customer oldCustomer)
{
SampleDBEntities dataContext = new SampleDBEntities();
var query = (from customer in dataContext.Customers
where customer.CustomerID==oldCustomer.CustomerID
select customer).SingleOrDefault();
query.CustomerID = oldCustomer.CustomerID;
query.ContactName = oldCustomer.ContactName;
query.City = oldCustomer.City;
dataContext.SaveChanges();
}
public static void DeleteCustomer(Customer oldCustomer)
{
SampleDBEntities dataContext = new SampleDBEntities();
dataContext.Customers.Remove(oldCustomer);
dataContext.SaveChanges();
}
}
Next we use the repository in our Controller as shown below –
public class CustomersController : ApiController
{
public List<Customer> Get()
{
return CustomersRepository.GetAllCustomers();
}
public Customer Get(string id)
{
return CustomersRepository.GetCustomer(id);
}
public void Post([FromBody]Customer customer)
{
CustomersRepository.InsertCustomer(customer);
}
public void Put([FromBody]Customer customer)
{
CustomersRepository.UpdateCustomer(customer);
}
public void Delete(string id)
{
CustomersRepository.DeleteCustomer(id);
}
}
Now let’s register the Web API actions with the route table. Let’s add “Global.asax” file and in the Application_Start method, add the following code –
protected void Application_Start(object sender, EventArgs e)
{
RouteTable.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = System.Web.Http.RouteParameter.Optional }
);
}
Now your Web API is ready to use. We will test this API using Fiddler.
It also supports traffic debug for the devices like Windows Phone, iPad/iPod and others. To download the Fiddler, go to www.fiddler2.com .

http://localhost:17019/api/customers
When you type the above address, it will select a Get () Method from our controller. The controller will give a call to repository logic which will fetch the data from SQL Server database using ADO.NET Entity Framework.
Return to fiddler and check the web sessions window. It will show you the result 200 which is a HTTP Status code for OK. On the right hand side, click on Inspectors tab and click on JSON at the bottom, you should see an output like below –
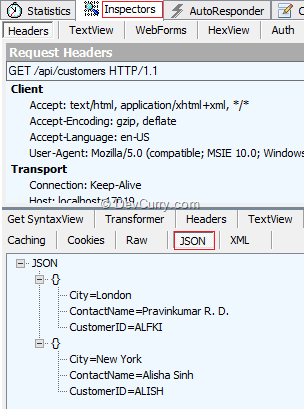
Next let’s try accessing the data using CustomerID which will fetch the Get (string id) method. This will fetch a specific record from our Customers table. Open Fiddler and click on Composer tab. Type below URI – http://localhost:17019/api/Customers/ALFKI and click on Execute button. You will see a similar output –
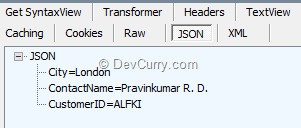
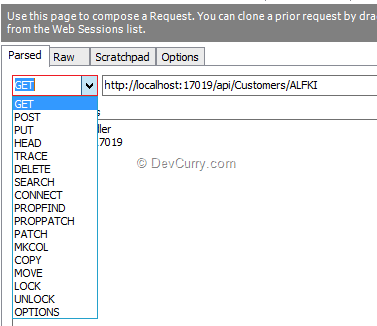
To perform the Insert operation using Fiddler, set the Verb to POST first. Then write the URI into our address bar. Specify the Content-Type : application/json. In the request body, write the following –
{"CustomerID":"JOHNR","ContactName":"John Rambo","City":"LONDON"}
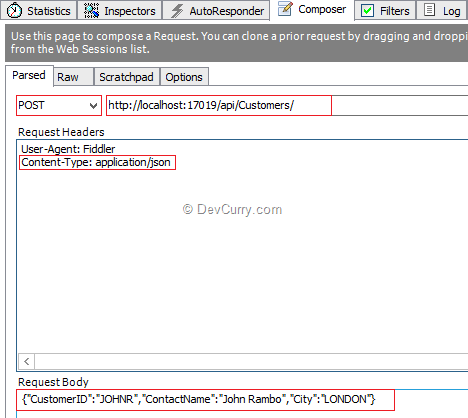
Now click on the Execute button. To Verify that data was saved, change the verb to GET and fetch all the customers. You will see the following output with the new record –
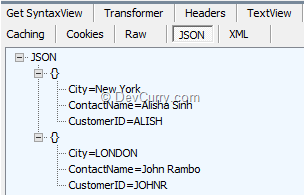
{"CustomerID":"JOHNR","ContactName":"John R.","City":"Mumbai"}
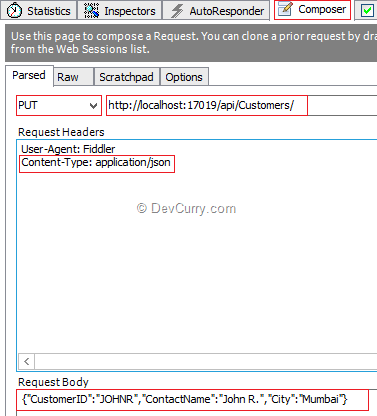
Fetch all the customers to see whether our update is successful or not.
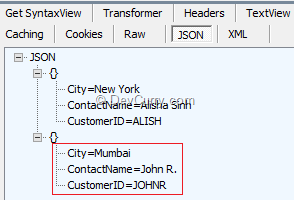
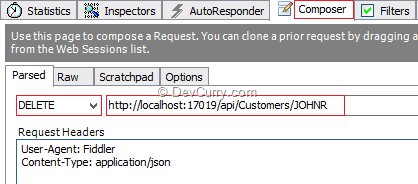
Now if you try to get the same record back, you will not see the record by using GET verb. This is how you can build ASP.NET Web API and test the same using Fiddler.
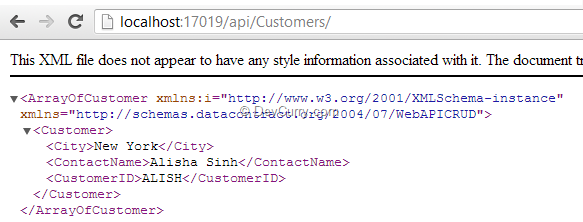
By using fiddler, you can change the serialization format by specifying the content-type as application/xml as shown below –
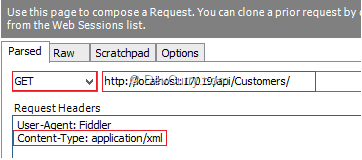
Now if you click on Inspectors tab, you see the output as shown below –
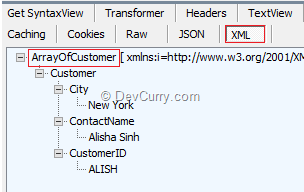
Download the entire source code of this article (Github)
When we think about exposing data on the web, we always talk about four common operations which we use in our day-to-day life –
1. Create
2. Retrieve/Read
3. Update
4. Delete
We call these operations as CRUD operations. HTTP provides 4 basic verbs which we can map to our CRUD operations as described below –
1. POST – CREATE
2. GET – RETRIVE
3. PUT – UPDATE
4. DELETE – DELETE
By exposing your services over plain HTTP, if you have any application that can connect to the web, they can consume your data. In Web API, when the data is pulled or pushed by using HTTP, the data is always serialized as JSON (JavaScript Object Notation) or XML.
Building the Web API Demo Application
Now let’s start by creating a new Web Application with the name “WebAPICRUD”. Open Visual Studio 2012. Click on New Project. Select ASP.NET Empty Web Application template. Once the project is setup, we have to ensure the backing database is present. You can use the CreateDatabase.sql in the code repository to create the DB or connect to an existing database and create a table with the name “Customers”. We can use the following script on an existing database too.CREATE TABLE Customers
(
CustomerID VARCHAR(10) PRIMARY KEY,
ContactName VARCHAR(50),
City VARCHAR(50)
)
Update the ‘SampleDBEntities’ connection string to point to the correct DB Server and Instance.
Insert some dummy data that can be fetched. Once your table is ready, let’s add a new item in our Web Application. Right click the Web Application and add a new item which is “ADO.NET Entity Data Model” as shown below –
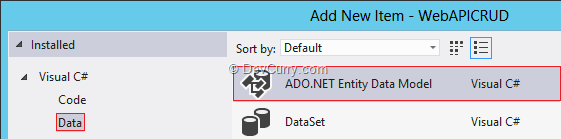
Now let’s follow the wizard steps. First Select “Generate from database”. Select connection string of your database. If the connection string is not available, create one and click on next button. Now in this step, Select Customers table from the Tables section and click on Finish button.
Now it’s time to implement our logic which will perform CRUD operations using ASP.NET Web API. When you are working with ASP.NET Web API, you will have to – Add a Web API Controller. Right click the ASP.NET Web Application and add a new Item. Select “Web API Controller Class”. Name it as “CustomersController” as shown below –
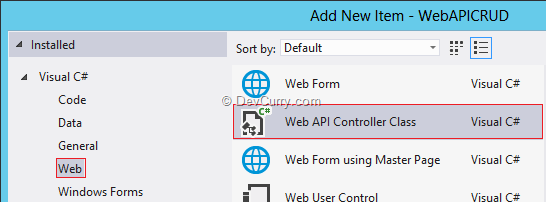
If you see, our “CustomersController” class is derived from ApiController class which belongs to System.Web.Http namespace as shown below –

This controller by default gives you the methods for your CRUD operations as described below –
1. Get () – Retrieve the data.
2. Get (int id) – Retrieve the data based on ID.
3. Post – Insert data.
4. Put – Update data.
5. Delete – Delete data.
Now let’s add a new class with the name “CustomersRepository” which will talk to our ADO.NET Entity framework which will then help our control to pull or push the data.
Once your class is ready, let’s add the following code to perform CRUD operations using our Entity Framework –
public class CustomersRepository
{
private static List<Customer> m_customersData;
public static List<Customer> GetAllCustomers()
{
SampleDBEntities dataContext=new SampleDBEntities();
var query = from customer in dataContext.Customers
select customer;
return query.ToList();
}
public static Customer GetCustomer(string customerID)
{
SampleDBEntities dataContext=new SampleDBEntities();
var query = (from customer in dataContext.Customers
select customer).SingleOrDefault();
return query;
}
public static void InsertCustomer(Customer newCustomer)
{
SampleDBEntities dataContext = new SampleDBEntities();
dataContext.Customers.Add(newCustomer);
dataContext.SaveChanges();
}
public static void UpdateCustomer(Customer oldCustomer)
{
SampleDBEntities dataContext = new SampleDBEntities();
var query = (from customer in dataContext.Customers
where customer.CustomerID==oldCustomer.CustomerID
select customer).SingleOrDefault();
query.CustomerID = oldCustomer.CustomerID;
query.ContactName = oldCustomer.ContactName;
query.City = oldCustomer.City;
dataContext.SaveChanges();
}
public static void DeleteCustomer(Customer oldCustomer)
{
SampleDBEntities dataContext = new SampleDBEntities();
dataContext.Customers.Remove(oldCustomer);
dataContext.SaveChanges();
}
}
Next we use the repository in our Controller as shown below –
public class CustomersController : ApiController
{
public List<Customer> Get()
{
return CustomersRepository.GetAllCustomers();
}
public Customer Get(string id)
{
return CustomersRepository.GetCustomer(id);
}
public void Post([FromBody]Customer customer)
{
CustomersRepository.InsertCustomer(customer);
}
public void Put([FromBody]Customer customer)
{
CustomersRepository.UpdateCustomer(customer);
}
public void Delete(string id)
{
CustomersRepository.DeleteCustomer(id);
}
}
Now let’s register the Web API actions with the route table. Let’s add “Global.asax” file and in the Application_Start method, add the following code –
protected void Application_Start(object sender, EventArgs e)
{
RouteTable.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = System.Web.Http.RouteParameter.Optional }
);
}
Now your Web API is ready to use. We will test this API using Fiddler.
Fiddler - a quick Intro
Fiddler is a tool which is used for logging the traffic between client (your computer) and Internet. Fiddler allows you to inspect the traffic, set breakpoints, and fiddle with incoming or outgoing data. Fiddler can debug the traffic that supports a proxy, including Internet Explorer, Google Chrome, Safari, Mozilla, Opera and many more.It also supports traffic debug for the devices like Windows Phone, iPad/iPod and others. To download the Fiddler, go to www.fiddler2.com .
Using Fiddler to exercise our Web API
Once Fiddler has been downloaded and installed on your computer, let’s open Fiddler. It should look like below –
GETting Data and inspecting it in Fiddler
Let’s open Internet Explorer and type this address -http://localhost:17019/api/customers
When you type the above address, it will select a Get () Method from our controller. The controller will give a call to repository logic which will fetch the data from SQL Server database using ADO.NET Entity Framework.
Return to fiddler and check the web sessions window. It will show you the result 200 which is a HTTP Status code for OK. On the right hand side, click on Inspectors tab and click on JSON at the bottom, you should see an output like below –
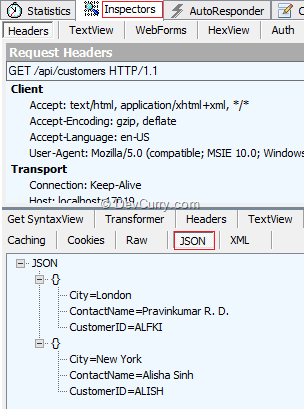
Next let’s try accessing the data using CustomerID which will fetch the Get (string id) method. This will fetch a specific record from our Customers table. Open Fiddler and click on Composer tab. Type below URI – http://localhost:17019/api/Customers/ALFKI and click on Execute button. You will see a similar output –
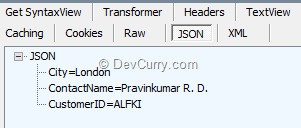
Creating records in DB by posting data through Fiddler
Open the Composer tab in Filddler. The default verb for HTTP is GET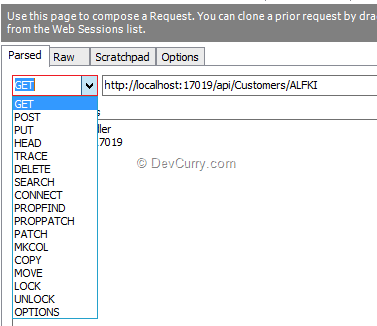
To perform the Insert operation using Fiddler, set the Verb to POST first. Then write the URI into our address bar. Specify the Content-Type : application/json. In the request body, write the following –
{"CustomerID":"JOHNR","ContactName":"John Rambo","City":"LONDON"}
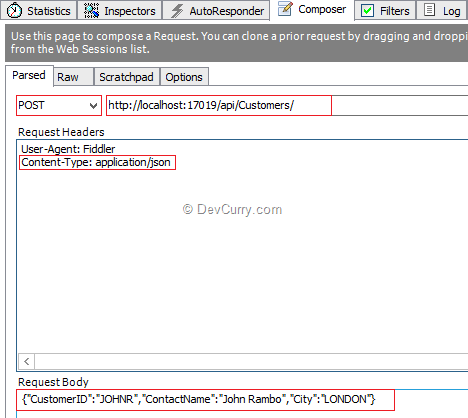
Now click on the Execute button. To Verify that data was saved, change the verb to GET and fetch all the customers. You will see the following output with the new record –
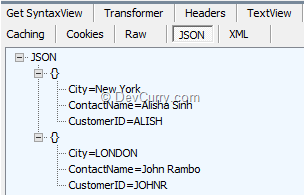
Updating Data using Web API by posting data via Fiddler
Now let’s try updating the data using fiddler. First of all set the Verb to PUT. Then write the URI into our address bar. Specify the Content-Type : application/json. In a request body, write the following –{"CustomerID":"JOHNR","ContactName":"John R.","City":"Mumbai"}
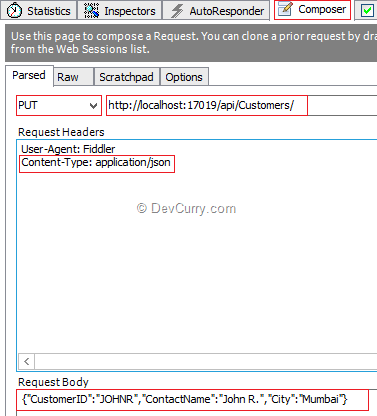
Fetch all the customers to see whether our update is successful or not.
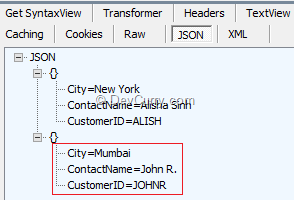
Deleting Data using Web API by posting request via Fiddler
Now let’s test the Delete operation using fiddler. Specify the URL - http://localhost:17019/api/Customers/JOHNR and change the verb to DELETE. Then click on Execute button as shown below –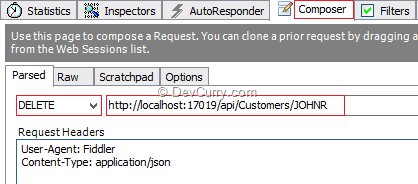
Now if you try to get the same record back, you will not see the record by using GET verb. This is how you can build ASP.NET Web API and test the same using Fiddler.
Fetching Data different Formats
Once last thing, if you fetch the data using Internet Explorer, the data is always serialized in JSON format. If you fetch the data using Google Chrome or Firefox, the data will always be serialized as XML as shown below –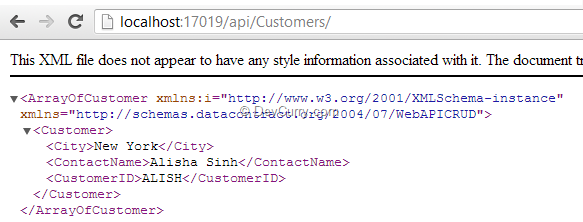
By using fiddler, you can change the serialization format by specifying the content-type as application/xml as shown below –
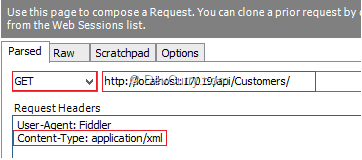
Now if you click on Inspectors tab, you see the output as shown below –
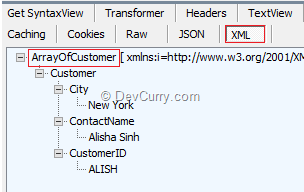
Summary
In this article, we have seen how to create ASP.NET Web API for performing CRUD operations and tested these operations using Fiddler tool. Note we did not build any UI and yet we were able to manage lifecycle of our data. This is the power of Web API services over HTTP.Download the entire source code of this article (Github)
Tweet
3 comments:
Nice and clear explanation.Thanks for the Author :)
can u plz explain what is "SampleDBEntities".Is it connection class???
Hi Good One,
But I am not getting by ID,Can you helpme?
Post a Comment