FlipView, represents an item control which displays one item at a time and enables user to use the flip behavior which is used for traversing through the collection of items. Typically such a control is used for traversing through Product Catalog, Books information etc. Technically the FlipView is a control provided in Windows Store Apps through Windows Library for JavaScript. The data to FlipView is made available through IListDataSource. (Note: You can get data from an external web sources like, web service, WCF service, WEB API in the JSON format).
We will use the FlipView for iterating through Images that’s passed to it from the WinJS List object.
Step 1: Open VS2012 and create a new Windows Store Apps using JavaScript. Name it as ‘Store_JS_FlipView’. In this project add some images in the ‘images’ folder. (I have some images as Mahesh.png, SachinN.png,SachinS.png, KiranP.jpg).
Step 2: In the default.html add the below Html code:
<div id="trgFilpView" data-win-control="WinJS.UI.FlipView"></div>
Note that the <div> is set to the WinJS.UI.FlipView using data-win-control property.
Step 3: Add the style for the FlipView in the default.html as below:
<style type="text/css">
#trgFilpView
{
width: 600px;
height: 500px;
border: solid 1px black;
background-color:coral;
}
</style>
Step 4: Since the FlipView accepts the IListDataSource, we need to define it as JSON data. To do this, add a new JavaScript file in the project, name it as ‘dataInformation.js’. Add the following code in it:
(function ()
{
"use strict";
//The JavaScript Array.
var trainerArray = [
{
name: "Sachin Shukre",
image: "images/SachinS.jpg",
description: "The Senior Corporate Trainer for C,C++"
},
{
name: "Mahesh Sabnis",
image: "images/Mahesh.png",
description: "The Senior Corporate Trainer for .NET"
},
{
name: "Sachin Nimbalkar",
image: "images/SachinN.jpg",
description: "The Senior Corporate Trainer for Client Side Frameworks"
},
{
name: "Mahesh Sabnis",
image: "images/KiranP.jpg",
description: "The Senior Corporate Trainer for C# and ASP.NET"
}];
//Define the List from the Array
var trainersList = new WinJS.Binding.List(trainerArray);
//This is the Private data
//To expose Data publically, define namespace which defines
//The object containing the Property-Value pair.
//The property is the public name of the member and the value is variable which contains data
var trainersInfo =
{
trList:trainersList
};
WinJS.Namespace.define("TrainersInformation", trainersInfo);
})();
The above code defines a JSON array with hard-coded data in it. This array is then passed to the ‘trainersList’ object defined as a List object using WinJS.Binding.List(). Since the array and the List are declared as private objects, these will not be exposed to the FlipView. To do this, we need to define the namespace which defines an object with property/value pair. The namespace is defined using WinJS.Namespace.define(). The ‘trList’ is the public property which contains the ‘trainersList’. This is now exposed to the FlipView.
Step 5: To display data into the FlipView, we need to define a Template for showing the repeated data. (Note: This is conceptually similar to templates in XAML). In the default.html add the below <div> tag, this is set to the WinJS.Binding.Template.
<!--Define the Template Here-->
<div id="DataTemplate" data-win-control="WinJS.Binding.Template">
<div>
<img src="#" data-win-bind="src : image"
style="width:100%;height:100%"/>
<div>
<h3 data-win-bind="innerText : name" ></h3>
<h4 data-win-bind="innerText : description" ></h4>
</div>
</div>
</div>
<!--Ends Here-->
The above template contains <img> which is bound to the ‘image’ property. which is coming from the List which is defined in Step 4 using the Array. The header <h3> and <h4> are used for displaying ‘name’ and ‘description’ declared in the array.
Step 6: To display the data in the FlipView, change the <div> with id ‘trgFlipView’ as shown below:
<div id="trgFilpView"
data-win-control="WinJS.UI.FlipView"
data-win-options=
"{
itemDataSource:TrainersInformation.trList.dataSource,
itemTemplate:DataTemplate
}">
</div>
To connect to the data, the itemsDataSource property of the FlipView is used. This property is assigned to trList object defined in the namespace in Step 4. The ItemTemplate property is set to the DataTemplate defined in Step 5.
Run the application, you will get the First Record from the Array.
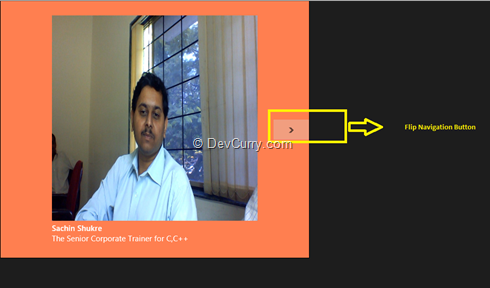
Click on the Flip Navigation button, the next record will be displayed:
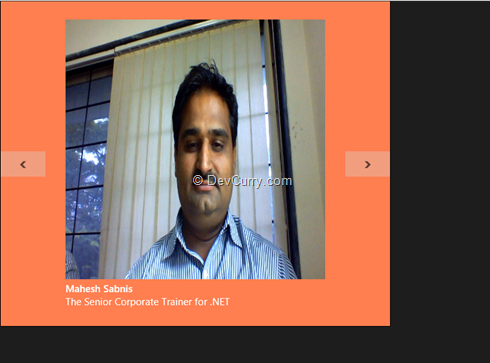
Conclusion
We saw how to bind an array of images to a FlipView control in WinJS. We can use the FlipView control to display any page-wise data, for example magazines, books etc.The entire source code of this article can be downloaded at https://github.com/devcurry/winrt-flipview
Tweet
No comments:
Post a Comment