Setting up NUnit
There are two parts to NUnit setup for use in Visual Studio. First part is to install the framework, we can do this in two ways.1. Download the 2.6.2 (latest at the time of writing) msi installer from http://www.nunit.org. Ensure Visual Studio is not running while the installer is running. This installs NUnit globally along with the NUnit test runner. However the test runner needs .NET 3.5 to run.
2. Another way to include NUnit in your project is to download it using the following Nuget Package Manager command
PM> install-package NUnit
Second part is to setup the NUnit Test Adapter so that Visual Studio recognizes the Test Cases in our project and allows us to Run them from the Test Explorer.
Setting up the NUnit Test Adapter
1. To Setup the adapter, go to Visual Studio Tools->Extensions and Updates.2. Select, Online in the Left hand pane Search for NUnit and Install the NUnit Test Adapter.
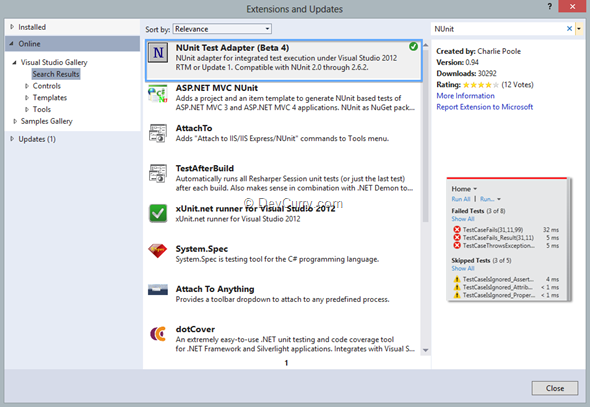
3. It needs a Visual Studio Restart
With that we are ready to write Test Cases using NUnit. So let’s write a sample Test Case.
Writing Test Cases for ASP.NET MVC Apps
Let’s create a new MVC4 project. The NUnit Test Adapter doesn’t register itself to be used with the ‘New Project Template’ dialog hence while creating the project, we can’t select NUnit. So we’ll not create a Test Project here. As shown below, if we select ‘Create a unit Test project’ NUnit is not registered as a Test Framework so we’ll uncheck the ‘Create a unit Test Project’ and continue.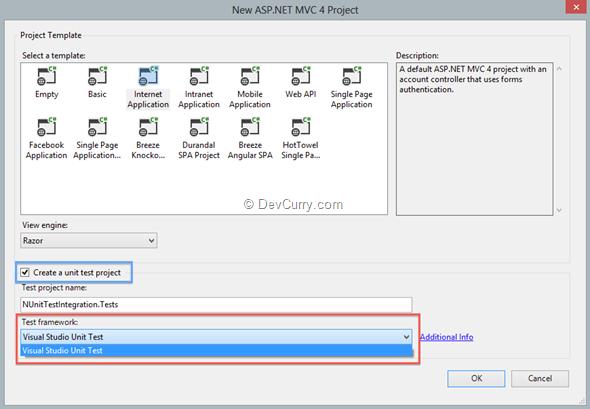
Once the MVC Project has been created, we add a simple Class Library project to the Solution and append the word ‘Test’ at the end to indicate it’s a Test project.
Next we add references to the Project using the Nuget Package Manager.
PM> install-package NUnit
Make sure you select the Class library in the Package Manager console.
Adding your first Test case
1. Rename the default Class1.cs to HomeControllerTest.2. Next add reference to System.Web.Mvc in the Test Project
3. Add a new Test case to test if view return has the name “Home”.
[TestFixture]
public class HomeControllerTest
{
[Test]
public void IndexActionReturnsIndexView()
{
string expected = "Index";
HomeController controller = new HomeController();
var result = controller.Index() as ViewResult;
Assert.AreEqual(expected, result.ViewName);
}
}
4. Build the project
5. Open Test Explorer from the ‘Test’ Menu.
6. The Test Explorer will scan all the projects for Test cases, you’ll see a green progress bar spinning on top. Once it completes, it will show the Test Case you just created.
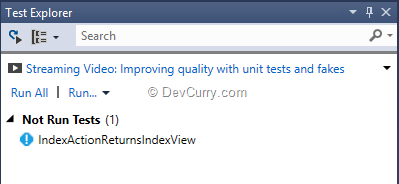
7. Run the Test case. It will fail. This is because the View name is not set and thus empty.
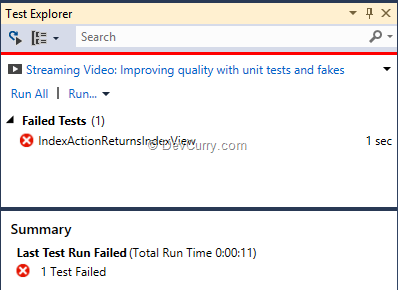
8. Go to the HomeController’s Index method and change the return statement to explicitly specify the ViewName.
public ActionResult Index()
{
ViewBag.Message = "Modify this template to jump-start your ASP.NET MVC application.";
return View("Index");
}
9. Build and Run the Test again. This time the test will succeed.
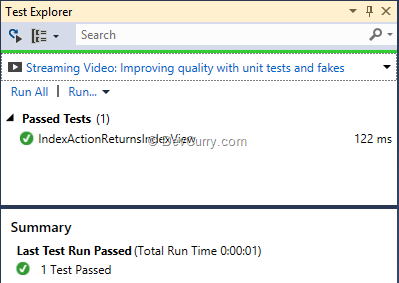
Conclusion
We saw how to setup NUnit and use the integration points in Visual Studio to integrate the Test case execution with Visual Studio’s tooling.Tweet
No comments:
Post a Comment