Here are some different ways to swap variable values in JavaScript. I will show swapping using a temporary variable, without a temporary variable, XOR operator and a single line swap.
Swap variable values using a temporary variable
// Using a Temp Variable var x = 10; var y = 20; var tmp = x; x = y; y = tmp; alert("Value of X=" + x + " and value of Y=" + y);
Swap variable values without a temporary variable
// Without using a temp variable var x = 10; var y = 20; x = x + y; y = x - y; x = x - y; alert("Value of X=" + x + " and value of Y=" + y);
Swap using Bitwise XOR operator
// Bitwise Exclusive OR operator var x = 10; var y = 20; x ^= y; y ^= x; x ^= y; alert("Value of X=" + x + " and value of Y=" + y);
Single line Swap (works in Firefox)
// JavaScript 1.7 and above var x = 10; var y = 20; [x, y] = [y, x]; alert("Value of X=" + x + " and value of Y=" + y);
OUTPUT
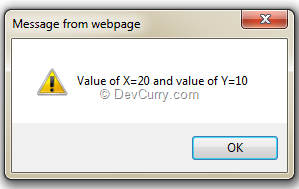
If you know of any other method to swap variable values in JavaScript, please share it with fellow readers.
Tweet
2 comments:
y = [x, x= y][0];
simpler..:)
thank u blogger
Post a Comment