The ASP.NET pipeline allows HTTP modules to be plugged-in to a request and intercept or modify each individual request. Modules can be used for processes like caching, authentication etc. However a basic requirement for an HTTP module to function, is that it must be registered in your config file. This leads to editing the Web.Config whenever you have to add/remove modules. I hate fudging with my config file too often!
Not known to many developers, ASP.NET 4.0 provides the PreApplicationStartMethodAttribute which allows you to run code even before any app_start event gets fired or any dynamic compilation occurs (App_code).
So how do I register an HTTP Module at Runtime using PreApplicationStartMethodAttribute and DynamicModuleUtility.RegisterModule?
It’s a simple 3 process step!
Step 1: Implement your Module. In the code shown below, we are implementing the IModule interface and subscribing to the BeginRequest event of the HttpApplication object. The OnBeginRequest method hooks up to the BeginRequest event.
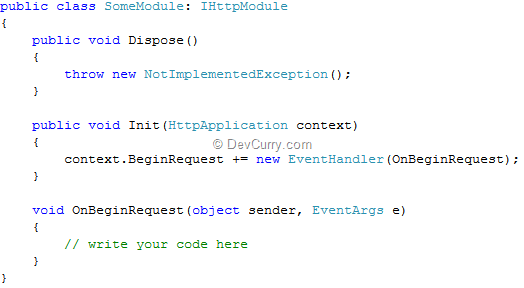
Step 2: Register the Module dynamically using the DynamicModuleUtility.RegisterModule
method. Write this code in the same class you created abovepublic static class MyCustomCode { public static void Initialize() { DynamicModuleUtility.RegisterModule(typeof(SomeModule)); } }
Step 3: The final step is to use the PreApplicationStartMethod attribute. Just add this attribute at the assembly level in the AssemblyInfo file or as shown below:

There you go! You have just registered an HTTP module into the ASP.NET pipeline without making any changes to web.config file.
Liked this tip? Check some more ASP.NET Tips here
Tweet
4 comments:
MSDN says:
DynamicModuleUtility.RegisterModule Method
This API supports the .NET Framework infrastructure and is not intended to be used directly from your code.
Interesting post but people shouldn't be telling readers to use PreApplicationStartMethod directly! You're limited to one of those for your entire App Domain so stealing it is criminal in an application.
Don't use PreApplicationStartMethod. Use WebActivate
Install-Package WebActivator
dotnetchris: Yes using WebActivator (https://bitbucket.org/davidebbo/webactivator/overview) is a nice alternative to overcome the limitation of a single instance of the attribute within each dll. Nice tip!
I have got to use those NuGet modules more often!
If class SomeModule has DI then how to register it.
Post a Comment