In this article, we will see how to bind Anonymous types with Silverlight 4.0 controls. For this demonstration, we will use the Silverlight ‘Datagrid’ control and also some LINQ.
Let us first create a Silverlight application as shown below:
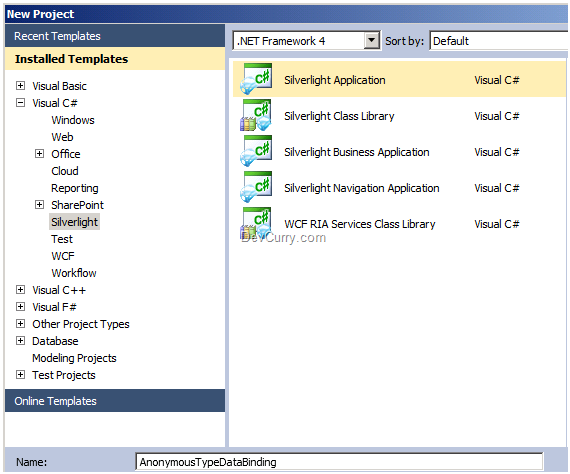
Once you click the ‘OK’ button, you will be given an option to host the Silverlight application in a new web site. Choose web site from ‘New Web Project Type’ as shown below –
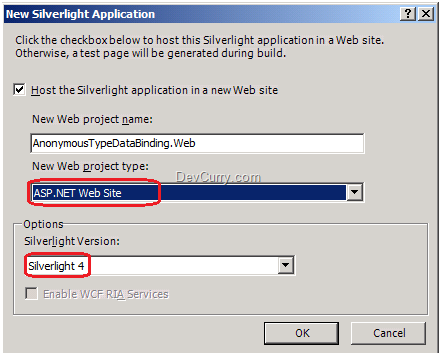
Now add two classes to the Silverlight application as shown below –
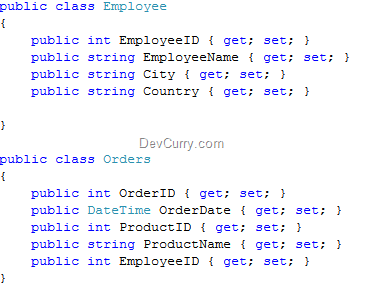
By default in Silverlight, you cannot bind ‘Anonymous type’ to Silverlight controls. The reason is ‘Anonymous types’ are generated in an assembly as internal types. But their exists a hack! To expose the internals of an assembly, add the following attribute to the ‘AssemblyInfo.cs’ file of Silverlight application as shown below –
[assembly: System.Runtime.CompilerServices.InternalsVisibleTo("System.Windows")]
Now drag and drop a ‘Datagrid’ on your Silverlight page as shown below –
<sdk:DataGrid AutoGenerateColumns="True" Height="243" HorizontalAlignment="Left" Margin="0,22,0,0" VerticalAlignment="Top" Width="400" x:Name="dgEmployee" />
Now in the code behind, declare two observable collections as shown below (make sure you are using the System.Collections.ObjectModel namespace) –
ObservableCollection<Employee> empCollection; ObservableCollection<Orders> orderCollection;
In the constructor of the Silverlight ‘MainPage’, initialize the collections and add few objects to the collections as shown below –
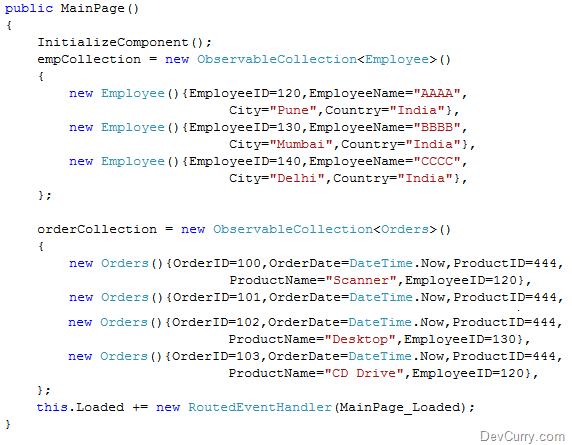
Now in the page load event, write the following query –
var query = from employees in empCollection join orders in orderCollection on employees.EmployeeID equals orders.EmployeeID select new { City = employees.City, Country = employees.Country, OrderID=orders.OrderID, OrderDate=orders.OrderDate, ProductName=orders.ProductName };
And now bind the datagrid to our anonymous type as shown below –
dgEmployee.ItemsSource = query;
Now run the application and you will see the Datagrid gets populated with the anonymous type data, as shown below –
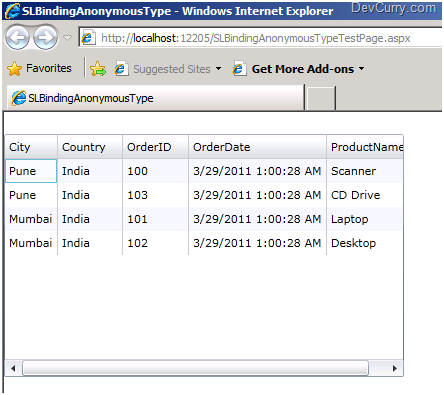
Note: I demonstrated a hack to bind Silverlight controls with ‘Anonymous Type’. So use it with caution!
Tweet
1 comment:
Thanks! I was wondering why this doesn't work in silverlight, will it work in WPF without the hack though? I am thinking it should but i am not sure.
Post a Comment