Here’s how to concatenate unique elements of two List<String> and then Sort them using LINQ. Use the Enumerable.Concat method
static void Main(string[] args) { List<string> lstOne = new List<string>() { "Jack", "Henry", "Amy" }; List<string> lstTwo = new List<string>() { "Hill", "Amy", "Anna" }; IEnumerable<string> lstNew = null; // Concatenate Unique Elements of two List<string> lstNew = lstOne.Concat(lstTwo).Distinct().OrderBy(x => x); PrintList(lstNew); Console.ReadLine(); } static void PrintList(IEnumerable<string> str) { foreach (var s in str) Console.WriteLine(s); Console.WriteLine("-------------"); }
VB.NET
Sub Main() Dim lstOne As New List(Of String)() From {"Jack", "Henry", "Amy"} Dim lstTwo As New List(Of String)() From {"Hill", "Amy", "Anna"} Dim lstNew As IEnumerable(Of String) = Nothing ' Concatenate Unique Elements of two List<string> lstNew = lstOne.Concat(lstTwo).Distinct().OrderBy(Function(x) x) PrintList(lstNew) Console.ReadLine() End Sub Shared Sub PrintList(ByVal str As IEnumerable(Of String)) For Each s In str Console.WriteLine(s) Next s Console.WriteLine("-------------") End Sub
OUTPUT
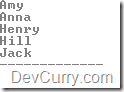
As you can see, Amy is not repeated twice as we called the IEnumerable.Distinct of the concatenated list.
Read some more tips in my article over here Some Common Operations using List<string>
Tweet
No comments:
Post a Comment