In this article, I will show you a simple way to validate a form. For this purpose, we will use a plugin called Bootstrap Validator (project on http://bootstrapvalidator.com/ ).
This plugin is based on Twitter Bootstrap.js and offers some great and expanding list of features. Some of them are:
- Bootstrap UI and web-fonts integration
- Validator initialization based on plugin options or HTML data-attribute
- Some built-in fields validators like: length of the content, date, credit card, IBAN, IMEI, phone, and some others
- Custom fields validators
- Possibility to add multiple validators to each field
- Possibility to show a feedback icon on the field after its validation
- Possibility to show the validation messages in a specific HTML element
- Rich API’s to manipulate the plugin instance and behaviors
- Some supported languages for localization
Bootstrap Validator Implementation
To use Bootstrap Validator, we first need to add the following dependencies in our project:- jQuery
- Bootstrap (js and css)
Here are the dependencies included (from CDN):
<head> <meta http-equiv="content-type" content="text/html; charset=UTF-8"> <title>Boostrap Validator</title> <script type="text/javascript" src="http://code.jquery.com/jquery-1.10.2.js"></script> <script src="http://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/js/bootstrap.min.js"></script> <link href="http://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css" rel="stylesheet"> <link rel="stylesheet" href="http://cdnjs.cloudflare.com/ajax/libs/jquery.bootstrapvalidator/0.5.0/css/bootstrapValidator.min.css"/> <script type="text/javascript" src="http://cdnjs.cloudflare.com/ajax/libs/jquery.bootstrapvalidator/0.5.0/js/bootstrapValidator.min.js"> </script> </head>
<form id="contactForm" method="post" class="form-horizontal"> <div class="form-group"> <label class="col-md-3 control-label">Full name</label> <div class="col-md-6"> <input type="text" class="form-control" name="fullName" /> </div> </div> <div class="form-group"> <label class="col-md-3 control-label">Email</label> <div class="col-md-6"> <input type="text" class="form-control" name="email" /> </div> </div> <div class="form-group"> <label class="col-md-3 control-label">Title</label> <div class="col-md-6"> <input type="text" class="form-control" name="title" /> </div> </div> <div class="form-group"> <label class="col-md-3 control-label">Content</label> <div class="col-md-6"> <textarea class="form-control" name="content" rows="5"></textarea> </div> </div> <!-- #messages is where the messages are placed inside --> <div class="form-group"> <div class="col-md-9 col-md-offset-3"> <div id="messages"></div> </div> </div> <div class="form-group"> <div class="col-md-9 col-md-offset-3"> <button type="submit" class="btn btn-default">Validate</button> </div> </div> </form>
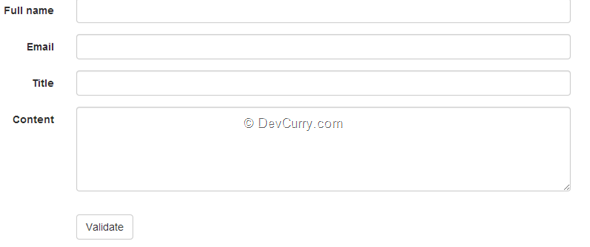
Now enable form validation. The code is self-explanatory:
$(document).ready(function() { $('#contactForm').bootstrapValidator({ container: '#messages', feedbackIcons: { valid: 'glyphicon glyphicon-ok', invalid: 'glyphicon glyphicon-remove', validating: 'glyphicon glyphicon-refresh' }, fields: { fullName: { validators: { notEmpty: { message: 'The full name is required and cannot be empty' } } }, email: { validators: { notEmpty: { message: 'The email address is required and cannot be empty' }, emailAddress: { message: 'The email address is not valid' } } }, title: { validators: { notEmpty: { message: 'The title is required and cannot be empty' }, stringLength: { max: 100, message: 'The title must be less than 100 characters long' } } }, content: { validators: { notEmpty: { message: 'The content is required and cannot be empty' }, stringLength: { max: 500, message: 'The content must be less than 500 characters long' } } } } }); });
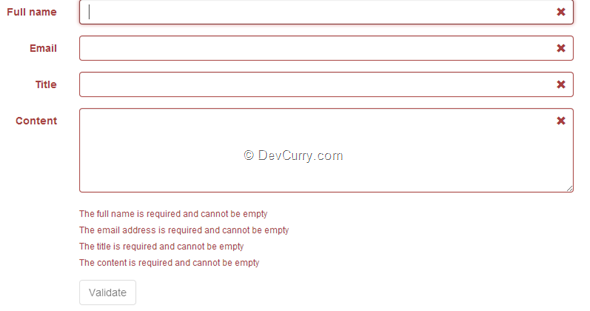
You will also observe that as we fill in the details, the valid fields turn green, thereby giving an immediate feedback to users.
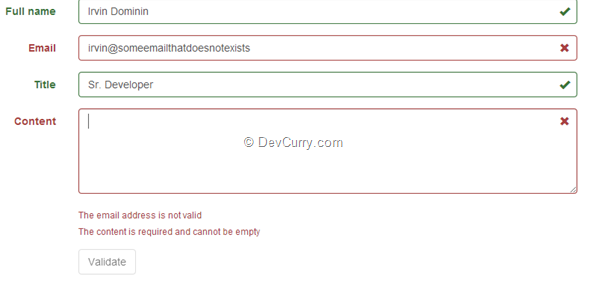
And that’s it. This way, the form will not be submitted until the required/validated fields are correctly filled.
Never forget to validate the submitted data to server. Also remember to perform server-side validation in addition to client side validation as JavaScript can be turned off by the user, there by bypassing the validation on client-side!
Tweet
9 comments:
Hello Sr, Thanks for the example.
I would like to ask you something :) my cuestion is: : I remember once time I used jquery.validator and now I see there is a new validator for bootstrap, and the sintax looks similar.
Would it be worth use bootstrap validator? Even when maybe someday we will apply some unobstrusive validations? and there is jquery.validator?
sir...i have a doubt that can bootstrap be validated using jsp's and servlets for server side validation
@Panzergrenadier sorry for the delay. They are different, but as you see they are very similar :-) Bootstrap validator is better in a bootstrap UI scenario. I never tried to mix validators, so I think will be better to use one technology a time in a page.
Is it possible to upload a file type using this bootstrap Validator? if possible, please can you give an example
@Sokoya Philip,
do you mean check file type in an input type file using bootstrap validation?
Hello,
it's really nice!
so.. how then I send the validated data to the server ?
thnks!
@Aylen Ricca You can call a server side script in the "success.form.fv" event, you take a look at the example here: http://formvalidation.io/examples/ajax-submit/
Dear sir
Thanks for example, I understand, I use it and works fine!!!! it is difficult to find good examples in internet and yours is a great contribution, again thanks
Jodocha from Venezuela
I need to add fields to validate dynamically so I would like to be able to set a java script variable with the validation fields and use that in the fields definition, I am not sure how to go about this
e.g.
var validationFields =
"\ta47: {\r\n" +
"\t\tvalidators: {\r\n" +
"\t\t\tnotEmpty: {\r\n" +
"t\t\t\tmessage: 'The Tickle Note is required and cannot be empty'\r\n" +
"\t\t\t},\r\n" +
"\t\t\temailAddress: {\r\n" +
"t\t\t\message: 'The email address is not valid'" +
"\t\t\t}\r\n" +
"\t\t}\r\n" +
"\t}\r\n";
$("#MainFormData").bootstrapValidator({
container: '#messages',
feedbackIcons: {
valid: 'glyphicon glyphicon-ok',
invalid: 'glyphicon glyphicon-remove',
validating: 'glyphicon glyphicon-refresh'
},
fields: {
validationFields
}
Post a Comment