Here, MembershipProvider is useful for large applications but there are some serious limitations of this approach. Maintaining the user’s security information is a very big responsibility and even though passwords are saved as salted hashes, the scenario of the entire database being compromised cannot be overlooked. Also the fact that every website requiring the user to register before using the application becomes irritating for the end-user because it is difficult for them to remember passwords, secret questions, etc. Often users ignore content that’s behind a mandatory authentication firewall. So now what’s the solution?
This is where OAuth and OpenID come in. These mechanisms allow the end-user to make use of their existing accounts on other trusted sites like Google, Microsoft, Yahoo to log on the site.
Previously setting OAuth and OpenID for a site was a little tedious. ASP.NET MVC 4 has made this easy by providing in-built support for OAuth and OpenID protocols. This support is provided using the DotNetOpenAuth library. This new support is by default available in the newly modified ‘AccountController’ class. As an MVC 4 developer, you can get the DotNetOpenAuth library using Nuget packages as shown below (Note: you don’t need to do this if you are using MVC Internet Web Application Template project to get started):
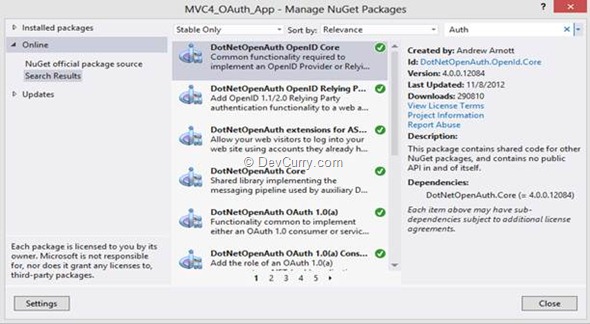
The AccountController class makes use of the ‘OAuthWebSecurity’ class to manage external logins. This class manages security that uses OAuth authentication providers like Windows Live, FaceBook, and OpenID authentication providers like Google.
Step 1: Open VS2012 and create a new MVC 4 application. Name it as ‘MVC4_OAuth_App’. Using Nuget Packages shown in the above screenshot, add the OpenAuth references in the project.
Step 2: Expand the ‘App_Start’ folder and open ‘AuthConfig.cs’ file. This is the ‘AuthConfig’ static class. This contains ‘RegisterAuth’ method. This method allows users to log on to this site using their accounts on Google, Facebook, and Windows Live etc. Here you will find some commented code.
Step 3: Uncomment the following line in the method:
OAuthWebSecurity.RegisterGoogleClient();
Step 4: Run the application and click on “Login”, the following result will be displayed:
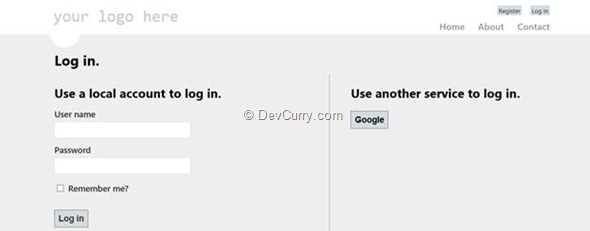
Click on the ‘Google’ button, it redirects to the Google confirmation page that verifies that you want to provide the information back to the requesting site, as shown below:
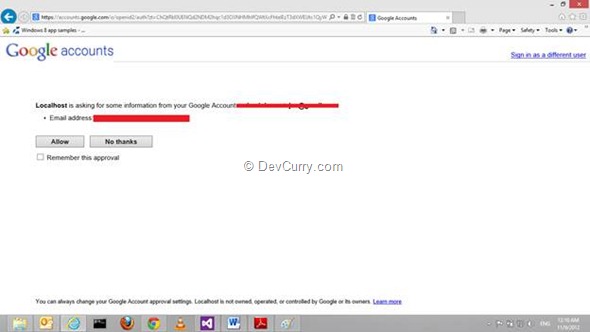
Click on ‘Allow’. Now you will be transferred to the Google login Page as below:
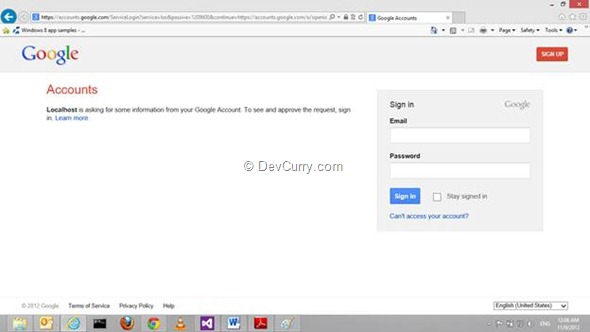
Enter the username and password, and click on Sign In. Here you will be asked to associate
the Google Account to the web site as shown below:
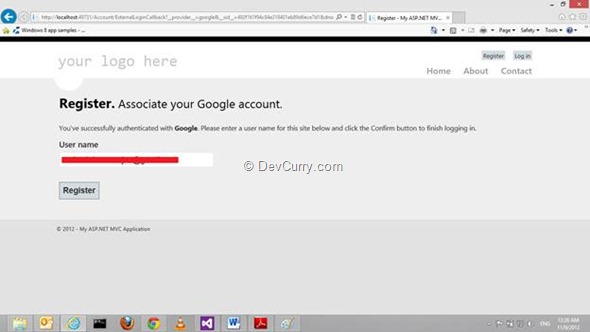
Click on the Register button and you will find the home page with the credentials:
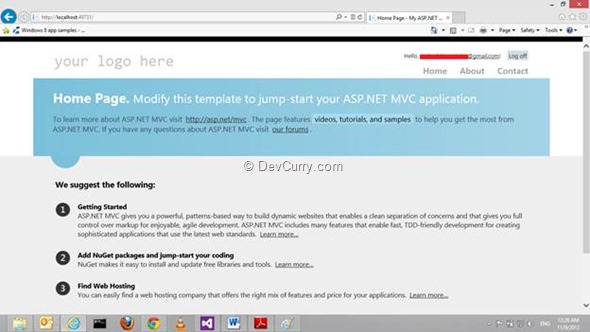
Note: Integration with Twitter or Facebook requires a few additional steps where you need to register with these sites to obtain correct tokens and provide them in your application. However that experience too is nearly code free.
Conclusion
With OAuth and OpenID, it’s easy for the developer to provide an external account login service to an internet facing web site. This reduces the overhead of having the application database storing secure information.Tweet
4 comments:
Thanks, nice write up, been meaning to try out OAuth for a while but heard it was tedious to set up. Guess not.
Hi Mahesh, Nice article once again. OpenID is good sign-in, but is there a way i can sign-out from external login.
Please do suggest, is there any other plug-in will do same as simple as this?
Try WebSecurity.Logout()
is this a valid solution for web developer express 2010? Thanks
Post a Comment